使用Word2vec和GloVe实现预训练词嵌入
时间: 2024-05-15 19:15:52 浏览: 11
预训练词嵌入是一种常见的自然语言处理技术,它将单词映射到向量空间中的点,使得单词在语义上相近的点在向量空间中也相近。
Word2vec和GloVe是两种常用的预训练词嵌入模型,可以通过使用Python中的gensim包来实现。
首先,我们需要准备一个文本语料库,可以是任何文本数据集。然后,我们可以使用gensim包中的Word2Vec和GloVe类来训练词嵌入模型。下面是一个简单的代码示例:
```
from gensim.models import Word2Vec
from gensim.scripts.glove2word2vec import glove2word2vec
from gensim.models import KeyedVectors
# 使用Word2vec训练词嵌入模型
sentences = [['this', 'is', 'the', 'first', 'sentence'],
['this', 'is', 'the', 'second', 'sentence'],
['yet', 'another', 'sentence'],
['one', 'more', 'sentence'],
['and', 'the', 'final', 'sentence']]
model_w2v = Word2Vec(sentences, size=100, min_count=1)
# 使用GloVe训练词嵌入模型
glove_input_file = 'glove.6B.100d.txt'
word2vec_output_file = 'glove.6B.100d.txt.word2vec'
glove2word2vec(glove_input_file, word2vec_output_file)
model_glove = KeyedVectors.load_word2vec_format(word2vec_output_file, binary=False)
# 使用训练好的模型进行单词相似度计算
similarity_w2v = model_w2v.wv.similarity('first', 'second')
similarity_glove = model_glove.similarity('first', 'second')
print('Word2vec similarity:', similarity_w2v)
print('GloVe similarity:', similarity_glove)
```
以上代码中,我们首先使用Word2vec训练了一个词嵌入模型,然后使用GloVe训练了另一个模型。我们还使用gensim包中的similarity函数计算了两个模型中单词“first”和“second”的相似度。
需要注意的是,GloVe模型在训练过程中需要使用预训练的GloVe向量文件作为输入,因此我们需要先将GloVe向量文件转换为Word2vec格式,然后再使用KeyedVectors类加载模型。
总的来说,Word2vec和GloVe是两种非常有用的预训练词嵌入技术,可以帮助我们更好地理解和处理自然语言数据。
相关推荐
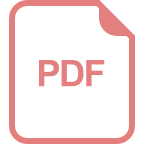
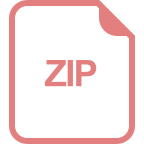
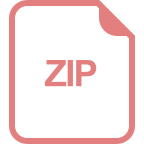
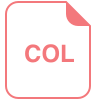
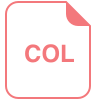
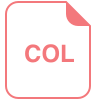











