写一个在jupyter notebooks上用RGB分量分别压缩对彩色图像进行图像压缩的代码,计算PSNR
时间: 2024-02-15 16:06:05 浏览: 39
好的,以下是一个在Jupyter Notebook上使用RGB分量对彩色图像进行压缩,并计算PSNR的代码:
```python
from skimage import io, color, img_as_float
import numpy as np
import math
# 读入彩色图像
img = io.imread('color_image.jpg')
# 将图像转换为浮点数类型
img = img_as_float(img)
# 分离RGB分量
r = img[:, :, 0]
g = img[:, :, 1]
b = img[:, :, 2]
# 对每个分量分别进行压缩
r_compress = some_function(r) # 这里需要根据你使用的压缩算法进行相应的操作
g_compress = some_function(g)
b_compress = some_function(b)
# 合并RGB分量
img_compress = np.zeros_like(img)
img_compress[:, :, 0] = r_compress
img_compress[:, :, 1] = g_compress
img_compress[:, :, 2] = b_compress
# 计算PSNR
mse = np.mean((img - img_compress) ** 2)
psnr = 10 * math.log10(1.0 / mse)
# 保存压缩后的图像
io.imsave('compressed_image.jpg', img_compress)
# 打印PSNR值
print('PSNR:', psnr)
```
在这个示例中,我们使用Scikit-image库中的`io.imread()`函数读入彩色图像,并使用`img_as_float()`函数将图像转换为浮点数类型。然后,我们使用NumPy库中的切片操作分离出RGB分量,并对每个分量分别进行压缩。在这里,你需要根据你使用的压缩算法进行相应的操作。最后,我们使用NumPy库中的数组操作将压缩后的RGB分量合并为一张彩色图像,并计算PSNR值。最后,我们使用`io.imsave()`函数保存压缩后的图像,并打印PSNR值。
请注意,这只是一个示例代码,你需要根据具体情况进行相应的修改和调整。
相关推荐
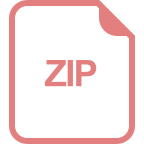
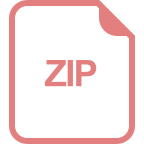
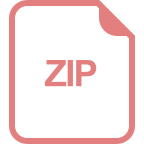














