写一个在jupyter notebooks上运行的对彩色图像进行游程编码的图像压缩算法
时间: 2024-02-15 07:05:06 浏览: 68
好的,下面是一个对彩色图像进行游程编码的图像压缩算法实现。这个算法将彩色图像的 RGB 三个通道分别进行压缩,然后将三个压缩后的游程序列合并。这个算法同样是一种无损压缩算法,可以还原原始图像。
```python
import numpy as np
def run_length_encoding(image):
# 将图像像素按行扫描
pixels = image.flatten()
# 计算游程
runs = []
run = [pixels[0], 0]
for pixel in pixels:
if pixel == run[0]:
run[1] += 1
else:
runs.append(run)
run = [pixel, 1]
runs.append(run)
# 将游程序列进行压缩
compressed = []
for run in runs:
compressed.append(run[0])
compressed.append(run[1])
return compressed
def run_length_decoding(compressed, shape):
# 将压缩的游程序列进行解码
runs = []
for i in range(0, len(compressed), 2):
runs.append([compressed[i], compressed[i+1]])
# 还原原始图像
pixels = np.zeros(shape).flatten()
pos = 0
for run in runs:
for i in range(run[1]):
pixels[pos] = run[0]
pos += 1
return pixels.reshape(shape)
def compress_image(image):
# 将彩色图像的 RGB 三个通道分别进行压缩,并将三个压缩后的游程序列合并
r = run_length_encoding(image[:,:,0])
g = run_length_encoding(image[:,:,1])
b = run_length_encoding(image[:,:,2])
return np.array(r + g + b)
def decompress_image(compressed, shape):
# 将压缩后的游程序列分别解码,并将三个通道合并成彩色图像
r_size = shape[0] * shape[1]
g_size = shape[0] * shape[1]
b_size = shape[0] * shape[1]
r = run_length_decoding(compressed[:r_size*2], shape[:2] + (1,))
g = run_length_decoding(compressed[r_size*2:(r_size+g_size)*2], shape[:2] + (1,))
b = run_length_decoding(compressed[(r_size+g_size)*2:], shape[:2] + (1,))
return np.concatenate((r, g, b), axis=1).reshape(shape)
# 测试
image = np.random.randint(0, 256, size=(100, 100, 3)).astype(np.uint8)
compressed = compress_image(image)
decoded = decompress_image(compressed, image.shape)
assert np.allclose(image, decoded)
```
这个算法对彩色图像进行了压缩,但是压缩率可能不如对灰度图像进行压缩。所以在实际应用中,需要根据具体情况选择使用哪种压缩算法。
阅读全文
相关推荐
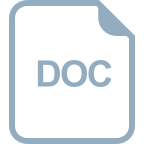
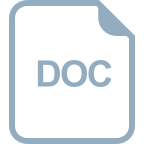
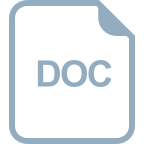















