retrofit发送POST请求@Body方式处理数据库参数
时间: 2023-05-30 09:07:59 浏览: 962
如果您使用Retrofit发送POST请求@Body方式处理数据库参数,可以按照以下步骤进行操作:
1. 创建一个Java类,用于存储参数。例如,如果您要向数据库添加一条用户记录,可以创建一个名为User的Java类,其属性包括用户名,密码等等。
```java
public class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
}
```
2. 在Retrofit接口中定义POST请求,使用@Body注解将User对象作为请求体发送到服务器。
```java
public interface ApiService {
@POST("users")
Call<Void> createUser(@Body User user);
}
```
3. 在应用中使用Retrofit对象创建接口实例,并调用createUser方法。
```java
// 创建Retrofit对象
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://example.com/api/")
.addConverterFactory(GsonConverterFactory.create())
.build();
// 创建接口实例
ApiService apiService = retrofit.create(ApiService.class);
// 创建User对象
User user = new User("username", "password");
// 发送POST请求
Call<Void> call = apiService.createUser(user);
call.enqueue(new Callback<Void>() {
@Override
public void onResponse(Call<Void> call, Response<Void> response) {
// 请求成功
}
@Override
public void onFailure(Call<Void> call, Throwable t) {
// 请求失败
}
});
```
上述代码将向服务器发送一条POST请求,将User对象作为请求体发送到服务器。如果请求成功,服务器将会将User对象存储到数据库中。如果请求失败,将会调用onFailure方法。
阅读全文
相关推荐
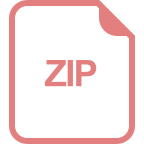















