使用c++实现机房收费管理系统
时间: 2023-09-21 20:07:51 浏览: 99
机房收费管理系统需要实现以下功能:
1. 学生信息管理:包括添加、删除、修改、查询学生信息等。
2. 机房设备管理:包括添加、删除、修改、查询机房设备信息等。
3. 收费管理:包括学生缴费、退费、查询学生缴费记录等。
4. 统计报表:包括每月学生缴费情况、机房设备使用情况等。
以下是一个简单的机房收费管理系统的代码示例:
```
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 学生信息类
class Student {
public:
Student(string name, int id) : name(name), id(id) {}
string getName() const { return name; }
int getId() const { return id; }
private:
string name;
int id;
};
// 机房设备类
class Equipment {
public:
Equipment(string name, int price) : name(name), price(price), status(false) {}
string getName() const { return name; }
int getPrice() const { return price; }
bool getStatus() const { return status; }
void setStatus(bool s) { status = s; }
private:
string name;
int price;
bool status;
};
// 收费记录类
class Payment {
public:
Payment(Student* s, Equipment* e, int amount) : student(s), equipment(e), amount(amount) {}
Student* getStudent() const { return student; }
Equipment* getEquipment() const { return equipment; }
int getAmount() const { return amount; }
private:
Student* student;
Equipment* equipment;
int amount;
};
// 学生信息管理类
class StudentManager {
public:
void addStudent(Student* s) { students.push_back(s); }
void removeStudent(Student* s) {
for (auto it = students.begin(); it != students.end(); it++) {
if (*it == s) {
students.erase(it);
break;
}
}
}
void modifyStudent(Student* s, string name) { s->name = name; }
Student* findStudentById(int id) {
for (auto it = students.begin(); it != students.end(); it++) {
if ((*it)->getId() == id) {
return *it;
}
}
return nullptr;
}
void printAllStudents() {
for (auto it = students.begin(); it != students.end(); it++) {
cout << "ID: " << (*it)->getId() << ", Name: " << (*it)->getName() << endl;
}
}
private:
vector<Student*> students;
};
// 机房设备管理类
class EquipmentManager {
public:
void addEquipment(Equipment* e) { equipments.push_back(e); }
void removeEquipment(Equipment* e) {
for (auto it = equipments.begin(); it != equipments.end(); it++) {
if (*it == e) {
equipments.erase(it);
break;
}
}
}
void modifyEquipment(Equipment* e, string name, int price) { e->name = name; e->price = price; }
Equipment* findEquipmentByName(string name) {
for (auto it = equipments.begin(); it != equipments.end(); it++) {
if ((*it)->getName() == name) {
return *it;
}
}
return nullptr;
}
void printAllEquipments() {
for (auto it = equipments.begin(); it != equipments.end(); it++) {
cout << "Name: " << (*it)->getName() << ", Price: " << (*it)->getPrice() << ", Status: " << ((*it)->getStatus() ? "In use" : "Available") << endl;
}
}
private:
vector<Equipment*> equipments;
};
// 收费管理类
class PaymentManager {
public:
void addPayment(Payment* p) { payments.push_back(p); p->getEquipment()->setStatus(true); }
void removePayment(Payment* p) {
for (auto it = payments.begin(); it != payments.end(); it++) {
if (*it == p) {
payments.erase(it);
p->getEquipment()->setStatus(false);
break;
}
}
}
void printAllPayments() {
for (auto it = payments.begin(); it != payments.end(); it++) {
cout << "Student: " << (*it)->getStudent()->getName() << ", Equipment: " << (*it)->getEquipment()->getName() << ", Amount: " << (*it)->getAmount() << endl;
}
}
int getTotalAmount() {
int total = 0;
for (auto it = payments.begin(); it != payments.end(); it++) {
total += (*it)->getAmount();
}
return total;
}
private:
vector<Payment*> payments;
};
// 统计报表类
class ReportManager {
public:
void printMonthlyReport() {
// TODO: 实现每月学生缴费情况统计报表
}
void printEquipmentUsageReport() {
// TODO: 实现机房设备使用情况统计报表
}
};
// 主函数
int main() {
StudentManager sm;
EquipmentManager em;
PaymentManager pm;
ReportManager rm;
// 添加学生
sm.addStudent(new Student("Tom", 1));
sm.addStudent(new Student("Jerry", 2));
// 添加机房设备
em.addEquipment(new Equipment("Computer", 1000));
em.addEquipment(new Equipment("Projector", 2000));
// 学生缴费
Student* s = sm.findStudentById(1);
Equipment* e = em.findEquipmentByName("Computer");
pm.addPayment(new Payment(s, e, 500));
// 查询学生缴费记录
pm.printAllPayments();
// 查询机房设备使用情况
em.printAllEquipments();
// 统计报表
rm.printMonthlyReport();
rm.printEquipmentUsageReport();
return 0;
}
```
以上代码只是一个简单的示例,实际应用中还需要根据具体需求进行修改和完善。
相关推荐
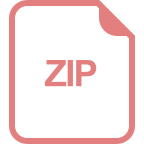
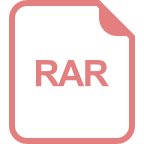
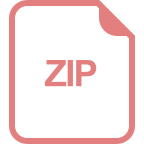













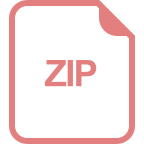
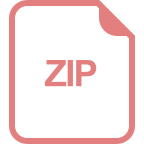