c# 反射设置类型为类的对象属性值
时间: 2024-09-25 22:02:55 浏览: 35
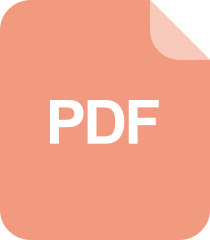
C#通过属性名字符串获取、设置对象属性值操作示例
在 C# 中,反射提供了一种动态访问程序运行时信息的方法,包括获取类型、方法、字段等。如果你想使用反射来设置类型为类的对象的属性值,你可以按照以下步骤操作:
1. **获取类型对象**:
首先,你需要获取你要操作的类的 `Type` 对象。如果你有一个已知的类型名称(如字符串),你可以用 `Type.GetType()` 方法获取。
```csharp
Type myClassType = Type.GetType("Namespace.ClassName");
```
2. **获取属性信息**:
使用 `GetProperty()` 方法找到指定名称的属性,如果属性存在,返回 `PropertyInfo` 对象。
```csharp
string attributeName = "PropertyName";
PropertyInfo property = myClassType.GetProperty(attributeName);
```
3. **检查属性可写性**:
在设置属性之前,确保属性是可以被写的(即它是可赋值的)。
```csharp
if (property != null && property.CanWrite)
{
// ...
}
else
{
Console.WriteLine($"无法写入属性 '{attributeName}'");
return;
}
```
4. **获取属性值(如果有的话)并设置新值**:
如果属性是可写的,你可以获取当前值并设置新的值。这里假设属性是 `public` 或者有合适的访问修饰符。
```csharp
object currentValue = property.GetValue(myObjectInstance); // 获取当前值
object newValue = ...; // 新的值
property.SetValue(myObjectInstance, newValue); // 设置新值
```
5. **处理异常**:
当尝试访问或修改属性时,可能会抛出异常,比如找不到属性或权限不足。记得捕获这些异常。
```csharp
try
{
// 设置属性值
}
catch(TargetInvocationException ex)
{
Console.WriteLine($"错误: {ex.InnerException.Message}");
}
catch(Exception ex)
{
Console.WriteLine($"错误: {ex.Message}");
}
```
阅读全文
相关推荐
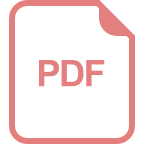
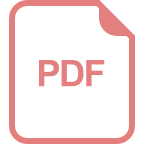















