linux读取串口数据
时间: 2023-06-05 19:47:50 浏览: 1276
Linux可以通过串口设备文件读取串口数据。首先需要确定串口设备文件的路径,一般为/dev/ttyS或/dev/ttyUSB等。然后使用文件读写函数(如open、read等)打开并读取该设备文件即可获取串口数据。需要注意的是,读取串口数据时需要设置正确的波特率、数据位、停止位、校验位等参数,以保证数据的正确性。
相关问题
linux读取串口数据read函数
在 Linux 中读取串口数据需要使用 read 函数,具体步骤如下:
1. 打开串口设备,可以使用 open 函数。
2. 配置串口,可以使用 tcsetattr 函数。
3. 循环读取串口数据,可以使用 read 函数,读取的数据将存储在缓冲区中。
4. 对读取的数据进行处理。
下面是一个简单的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <string.h>
#include <unistd.h>
#include <termios.h>
int main()
{
int fd; // 串口文件描述符
char buf[256]; // 缓冲区
int n; // 读取的字节数
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("open");
return -1;
}
// 配置串口
struct termios options;
tcgetattr(fd, &options);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &options);
// 循环读取串口数据
while (1) {
memset(buf, '\0', sizeof(buf));
n = read(fd, buf, sizeof(buf));
if (n > 0) {
printf("read %d bytes: %s\n", n, buf);
}
}
// 关闭串口设备
close(fd);
return 0;
}
```
需要注意的是,在读取串口数据之前,需要先配置串口参数,具体参数根据实际情况而定。另外,在读取数据时,需要指定最大读取字节数,否则可能会一直阻塞在 read 函数上。
LINUX C 打开 读取串口数据
在 Linux C 中打开并读取串口数据,可以使用以下步骤:
1. 打开串口设备文件,使用 open() 函数,例如:
```
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open error");
exit(1);
}
```
其中,/dev/ttyS0 表示串口设备文件路径,O_RDWR 表示读写方式打开,O_NOCTTY 表示不将串口设备作为控制终端,O_NDELAY 表示非阻塞方式打开。
2. 配置串口参数,使用 termios 结构体和 tcsetattr() 函数,例如:
```
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CRTSCTS;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
```
其中,B9600 表示波特率,CLOCAL 和 CREAD 表示忽略 modem 状态和启用接收器,PARENB 表示启用奇偶校验,CSTOPB 表示使用两个停止位,CSIZE 表示数据位数,CRTSCTS 表示启用 RTS/CTS 硬件流控,ICANON、ECHO、ECHOE、ISIG、OPOST 分别表示输入模式、回显、回显擦除、信号处理、输出模式,VMIN 和 VTIME 分别表示最小读取字符数和超时时间。
3. 读取串口数据,使用 read() 函数,例如:
```
char buf[1024];
int n = read(fd, buf, sizeof(buf));
if (n < 0) {
perror("read error");
exit(1);
} else if (n == 0) {
printf("no data\n");
} else {
printf("read %d bytes: %s\n", n, buf);
}
```
其中,buf 表示读取的数据缓冲区,sizeof(buf) 表示缓冲区大小,n 表示实际读取的字节数。
4. 关闭串口设备文件,使用 close() 函数,例如:
```
close(fd);
```
注意,在使用串口之前,可能需要使用 root 权限或者将用户添加到 dialout 用户组中,以获得串口读写权限。
阅读全文
相关推荐
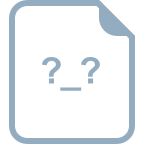
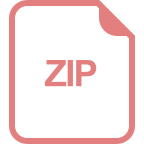
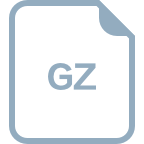
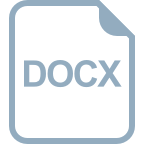
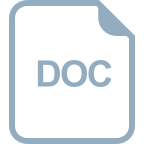
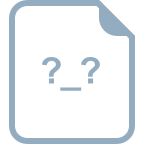
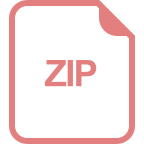
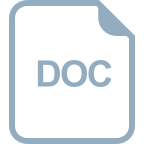



