linux 串口读取数据的示例
时间: 2023-12-01 16:00:52 浏览: 27
在Linux系统中,可以使用minicom工具来读取串口数据。首先,需要安装minicom工具,可以通过在终端输入以下命令进行安装:
```shell
sudo apt-get install minicom
```
安装完成后,可以通过以下步骤来读取串口数据:
1. 打开终端,输入以下命令来配置minicom:
```shell
sudo minicom -s
```
2. 在配置界面中,选择“Serial port setup”选项,设置串口参数,包括串口设备、波特率、数据位、停止位、校验位等参数。
3. 配置完成后,退出配置界面,返回终端界面。
4. 输入以下命令来打开串口:
```shell
sudo minicom -b 9600 -o -D /dev/ttyS0
```
其中,-b参数用于指定波特率,-o参数用于打开本地回显,-D参数后面跟着串口设备的路径。
5. 等待串口接收到数据后,可以在minicom界面上看到串口接收到的数据。
通过以上步骤,就可以在Linux系统中使用minicom工具来读取串口数据。需要注意的是,串口设备路径和波特率等参数需要根据实际情况进行设置,以确保正确读取串口数据。
相关问题
linux读取串口数据read函数
在 Linux 中读取串口数据需要使用 read 函数,具体步骤如下:
1. 打开串口设备,可以使用 open 函数。
2. 配置串口,可以使用 tcsetattr 函数。
3. 循环读取串口数据,可以使用 read 函数,读取的数据将存储在缓冲区中。
4. 对读取的数据进行处理。
下面是一个简单的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <string.h>
#include <unistd.h>
#include <termios.h>
int main()
{
int fd; // 串口文件描述符
char buf[256]; // 缓冲区
int n; // 读取的字节数
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("open");
return -1;
}
// 配置串口
struct termios options;
tcgetattr(fd, &options);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &options);
// 循环读取串口数据
while (1) {
memset(buf, '\0', sizeof(buf));
n = read(fd, buf, sizeof(buf));
if (n > 0) {
printf("read %d bytes: %s\n", n, buf);
}
}
// 关闭串口设备
close(fd);
return 0;
}
```
需要注意的是,在读取串口数据之前,需要先配置串口参数,具体参数根据实际情况而定。另外,在读取数据时,需要指定最大读取字节数,否则可能会一直阻塞在 read 函数上。
linux串口读写程序
以下是一个简单的Linux串口读写程序的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char *argv[]) {
int fd;
struct termios options;
char buffer[255];
// 打开串口
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
exit(1);
}
// 配置串口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &options);
// 写入数据
char *message = "Hello, world!";
write(fd, message, strlen(message));
// 读取数据
memset(buffer, 0, sizeof(buffer));
int n = read(fd, buffer, sizeof(buffer));
if (n > 0) {
printf("Received message: %s\n", buffer);
}
// 关闭串口
close(fd);
return 0;
}
```
该程序首先打开了 `/dev/ttyS0` 设备文件,然后配置了串口的波特率、数据位、校验位、停止位等参数。接着,程序向串口写入了一条消息,然后读取了串口返回的数据。最后关闭了串口。注意,程序中的错误处理非常简单,实际应用中应该根据具体需求进行完善。
相关推荐
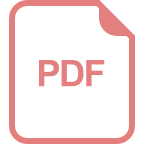
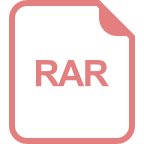
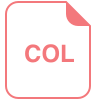
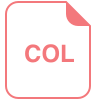
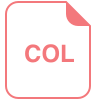
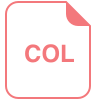
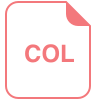







