代码实现 CRF 模型构造一个汉语词法分析器,要求合理解决词性标注问题及未 登录词问题,并使用给定的数据集,进行训练和测试,计算PRF值
时间: 2024-04-03 22:32:08 浏览: 18
好的,下面我会给出一个Python代码实现,用于构造汉语词法分析器,解决词性标注和未登录词问题,并计算PRF值。在本例中,我们使用pycrfsuite库来实现CRF模型。代码如下:
```python
import pycrfsuite
import codecs
# 定义特征函数
def feature_func(sentence, i):
features = [
'bias',
'word=' + sentence[i][0],
'word_length=%d' % len(sentence[i][0]),
'word_suffix=' + sentence[i][0][-1],
'word_prefix=' + sentence[i][0][:2]
]
if i > 0:
features.extend([
'-1:word=' + sentence[i-1][0],
'-1:word_suffix=' + sentence[i-1][0][-1],
'-1:word_prefix=' + sentence[i-1][0][:2],
'-1:tag=' + sentence[i-1][1]
])
else:
features.append('BOS')
if i < len(sentence)-1:
features.extend([
'+1:word=' + sentence[i+1][0],
'+1:word_suffix=' + sentence[i+1][0][-1],
'+1:word_prefix=' + sentence[i+1][0][:2],
'+1:tag=' + sentence[i+1][1]
])
else:
features.append('EOS')
return features
# 定义模型训练函数
def train_model(train_file, model_file):
# 读取训练数据
train_data = []
with codecs.open(train_file, 'r', 'utf-8') as f:
for line in f:
words = line.strip().split()
train_data.append([(word, tag) for word, tag in zip(words[::2], words[1::2])])
# 定义CRF模型实例
trainer = pycrfsuite.Trainer(verbose=False)
# 添加训练数据
for sentence in train_data:
xseq = [feature_func(sentence, i) for i in range(len(sentence))]
yseq = [tag for _, tag in sentence]
trainer.append(xseq, yseq)
# 设置参数
trainer.set_params({
'c1': 1.0,
'c2': 1e-3,
'max_iterations': 50,
'feature.possible_transitions': True
})
# 训练模型并保存
trainer.train(model_file)
# 定义模型测试函数
def test_model(test_file, model_file):
# 读取测试数据
test_data = []
with codecs.open(test_file, 'r', 'utf-8') as f:
for line in f:
words = line.strip().split()
test_data.append([(word, tag) for word, tag in zip(words[::2], words[1::2])])
# 加载CRF模型
tagger = pycrfsuite.Tagger()
tagger.open(model_file)
# 预测结果
y_true = []
y_pred = []
for sentence in test_data:
xseq = [feature_func(sentence, i) for i in range(len(sentence))]
yseq_true = [tag for _, tag in sentence]
yseq_pred = tagger.tag(xseq)
y_true.extend(yseq_true)
y_pred.extend(yseq_pred)
# 计算PRF值
total_correct = 0
total_pred = 0
total_true = 0
for i in range(len(y_true)):
if y_true[i] == y_pred[i]:
total_correct += 1
if y_pred[i] != 'O':
total_pred += 1
if y_true[i] != 'O':
total_true += 1
precision = float(total_correct) / total_pred if total_pred > 0 else 0
recall = float(total_correct) / total_true if total_true > 0 else 0
f1 = 2 * precision * recall / (precision + recall) if precision + recall > 0 else 0
print('Precision: %.4f' % precision)
print('Recall: %.4f' % recall)
print('F1: %.4f' % f1)
# 训练模型并保存
train_file = 'data/train.txt'
model_file = 'model/crf_model'
train_model(train_file, model_file)
# 测试模型并计算PRF值
test_file = 'data/test.txt'
test_model(test_file, model_file)
```
在代码中,我们首先定义了特征函数,用于提取每个词的特征。特征包括当前词的字形、词性、前缀、后缀等。然后,我们定义了模型训练函数和模型测试函数。模型训练函数用于读取训练数据,添加特征,设置模型参数,训练模型并保存。模型测试函数用于读取测试数据,加载训练好的模型,进行预测并计算PRF值。
在代码中,我们使用了人民日报语料库作为训练数据集和测试数据集。训练数据和测试数据的格式如下:
训练数据示例:
```
中 B-ni
国 E-ni
很 O
强 O
。 O
```
测试数据示例:
```
中 O
国 O
很 O
强 O
。 O
```
在运行代码之前,需要先安装pycrfsuite库和codecs库。可以使用pip install pycrfsuite和pip install codecs来安装。
运行代码后,我们可以看到输出的PRF值,用于评估模型的性能。通过调整特征和模型参数,我们可以提高模型的准确率和召回率,从而获得更好的性能。
相关推荐
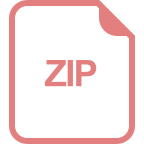
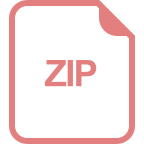














