不用pair结构,就用简单的比如排序算法来完成
时间: 2024-10-21 07:15:34 浏览: 8
如果你想不用pair直接使用排序算法,你可以先对所有点按照坐标(x, y)进行升序排序,然后遍历一次数组,给每个点分配排名。这里是一个示例,使用冒泡排序,因为它的复杂度较低:
```cpp
#include <iostream>
#include <vector>
struct Point {
int x;
int y;
};
bool comparePoints(const Point& a, const Point& b) {
return (a.x < b.x) || ((a.x == b.x) && (a.y < b.y));
}
void bubbleSort(std::vector<Point>& points) {
int n = points.size();
for (size_t i = 0; i < n - 1; ++i) {
for (size_t j = 0; j < n - 1 - i; ++j) {
if (comparePoints(points[j], points[j + 1])) {
// 交换点的位置
Point temp = points[j];
points[j] = points[j + 1];
points[j + 1] = temp;
}
}
}
}
int calculateRank(const std::vector<Point>& points) {
bubbleSort(points); // 先排序
int rank = 0;
for (const auto& point : points) {
if (rank == 0 || point != points[rank - 1]) rank++; // 如果当前点不同于前一个,排名加一
}
return rank;
}
int main() {
std::vector<Point> points = { ... }; // 这里同样需要替换为你实际的数据
int rank = calculateRank(points);
std::cout << "The rank of all points is: " << rank << std::endl;
return 0;
}
```
在这个版本中,我们首先使用冒泡排序对点进行排序,然后根据排序后的顺序计算排名。
阅读全文
相关推荐
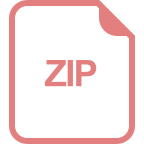
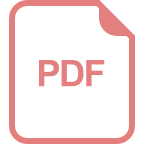
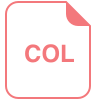
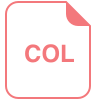
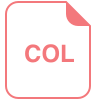
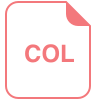
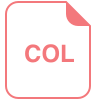
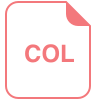
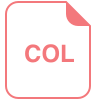
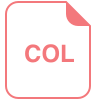
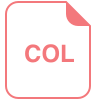
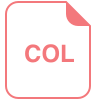
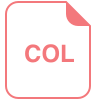
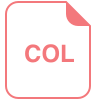
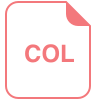
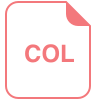
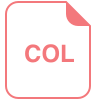
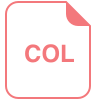
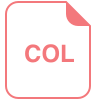