clion编写一个程序,在这个程序中完成如下功能: (1)建立顺序表。 (2)在顺序表上实现插入、删除和查找操作。 (3)实现遍历输出顺序表中所有元素。 (3)实现在有序顺序表中删除其中重复的元素,要求删除有序顺序表中重复元素的操作算法时间复杂度为
时间: 2024-10-08 22:06:12 浏览: 33
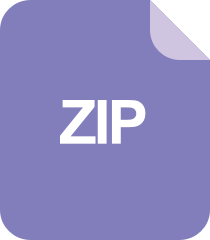
leetcode矩阵旋转任意角度-SwordRefersToOffer:剑指offer的C++代码,在ubuntu上CLion测试
在CLion中创建一个C++程序来实现这些功能,你可以按照以下步骤进行:
1. **建立顺序表**:
首先,你需要定义一个`Node`结构体表示顺序表中的节点,以及一个`SequentialList`类来存储节点并支持基本操作。
```cpp
struct Node {
int data;
Node* next;
};
class SequentialList {
private:
Node* head;
public:
// 构造函数和析构函数
SequentialList() : head(nullptr) {}
~SequentialList();
};
```
2. **插入操作**:
实现插入方法如`insert(int value)`,可以将新元素添加到链表的尾部。
```cpp
void SequentialList::insert(int value) {
if (!head) {
head = new Node{value, nullptr};
} else {
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = new Node{value, nullptr};
}
}
```
3. **删除操作**:
分别实现删除指定值的节点`deleteValue(int value)`和删除所有重复值的`removeDuplicates()`方法。
```cpp
bool removeDuplicate(Node*& node) {
if (!node) return false;
if (node->data == valueToBeRemoved) {
delete node;
node = node->next;
return true;
}
return removeDuplicate(node->next);
}
void SequentialList::removeDuplicates() {
Node* temp = head;
if (temp && temp->data == temp->next->data) {
delete temp;
temp = temp->next;
}
while (temp) {
if (removeDuplicate(temp)) {
temp = temp->next;
}
if (temp && temp->data == temp->next->data) {
delete temp->next;
}
}
}
```
4. **查找操作**:
添加`find(int value)`方法来查找特定值的位置。
```cpp
int SequentialList::find(int value) {
Node* current = head;
for (int i = 0; current && i < size(); ++i, current = current->next) {
if (current->data == value) return i;
}
return -1; // 如果未找到,则返回-1
}
```
5. **遍历输出**:
创建一个`display()`方法来打印顺序表的所有元素。
```cpp
void SequentialList::display() {
Node* current = head;
while (current) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << "\n";
}
```
6. **时间复杂度分析**:
插入、删除单个元素的时间复杂度都是O(1),因为它们都在链表头部或尾部操作。查找的时间复杂度取决于列表长度,为O(n)。删除所有重复项的时间复杂度也是O(n^2),因为在最坏的情况下,每个元素都需要遍历一次链表。
阅读全文
相关推荐
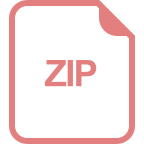
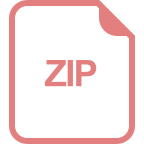
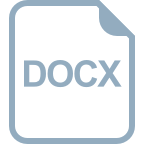
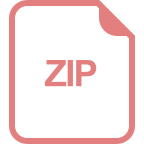
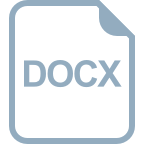
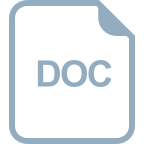
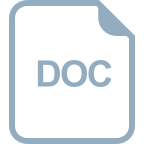
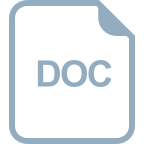
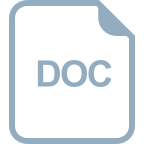
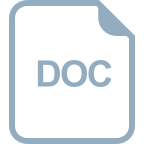
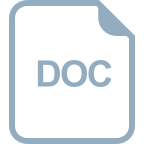
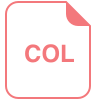
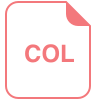
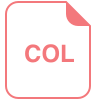
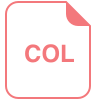
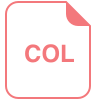
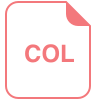
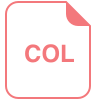