【反转链表不求人】:单向链表高效反转算法精讲
发布时间: 2024-09-11 12:58:54 阅读量: 115 订阅数: 24 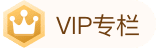
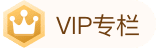

# 1. 链表基础与问题阐述
## 1.1 链表简介
链表是计算机科学中一种常见的基础数据结构,它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。链表的灵活之处在于它的动态内存分配和插入、删除操作的高效性。
## 1.2 链表类型
链表主要分为单向链表和双向链表。单向链表中的节点只包含一个指向下一个节点的指针,而双向链表的节点还包含一个指向前一个节点的指针。
## 1.3 链表问题提出
链表的挑战在于管理节点间的链接关系。一个常见的问题是反转链表,即将链表中所有节点的指向顺序颠倒,从左到右变为从右到左。
在本章中,我们将首先介绍链表的基本概念和结构,然后阐述反转链表的提出背景以及它在实际开发中的重要性和挑战。这为后面章节更深入地探讨反转链表的理论基础、实现方法和优化策略奠定基础。
# 2. 反转链表的理论基础
### 2.1 链表数据结构概述
#### 2.1.1 单向链表的基本概念
链表是一种常见的基础数据结构,它通过一系列的节点来存储数据,每个节点包含两部分信息:一部分是存储的数据,另一部分是指向下一个节点的指针(或称为引用)。在单向链表中,节点只能向一个方向遍历,即从头节点开始到尾节点结束。
单向链表的典型结构可以表示为:
```mermaid
classDiagram
class Node {
<<interface>>
data
next
}
class LinkedList {
head
}
Node "1" -- "*" LinkedList : uses >
```
在这个结构中,`LinkedList`类包含一个指向链表第一个元素(头节点)的指针`head`。`Node`类则定义了链表节点的数据部分`data`以及指向下一个节点的指针`next`。
单向链表具有以下特点:
- 动态大小:链表的大小不需要在创建时确定,可以根据需要动态地添加和删除节点。
- 插入和删除操作高效:在链表中插入或删除节点只需要改变相关节点的指针,不需要移动整个数据结构。
- 随机访问效率低:由于链表中的节点分散存储在内存中,访问链表中的某个位置需要从头节点开始遍历。
#### 2.1.2 链表节点的定义与指针操作
在不同的编程语言中,链表节点的实现会有所不同。以下是使用C语言和Python语言实现链表节点的示例。
C语言中定义一个简单的链表节点:
```c
struct Node {
int data;
struct Node* next;
};
```
在Python中,链表节点可以使用类来定义:
```python
class ListNode:
def __init__(self, value=0, next=None):
self.data = value
self.next = next
```
无论是哪种语言,实现节点时都需要注意以下几点:
- **内存分配**:动态分配节点的内存空间,确保内存的有效管理。
- **指针操作**:正确地操作节点的指针,特别是对`next`指针的赋值,以及处理头节点(可能需要特别对待)。
- **链表构建**:从头节点开始逐个构建链表,保证链表的完整性和节点间的正确连接。
- **内存释放**:在不再需要链表时,遍历链表并释放每个节点所占用的内存空间。
### 2.2 反转链表的算法思路
#### 2.2.1 算法问题的提出与初步分析
反转链表是一个常见的数据结构操作问题,其核心在于如何高效地将链表中的每个节点指针方向逆转,使得原本链表的最后一个节点变为第一个节点,原头节点变为尾节点。
问题提出:给定一个单向链表的头节点,编写算法实现链表的反转。
初步分析:要实现链表的反转,可以通过迭代的方式逐个调整节点的指针方向,或者使用递归的方式来简化操作。在迭代法中,需要维护三个指针,分别指向前一个节点、当前节点和下一个节点。在每次迭代中,将当前节点的指针方向反转,然后移动这三个指针。
#### 2.2.2 时间复杂度与空间复杂度的理解
时间复杂度:对于反转链表的操作,无论采取迭代法还是递归法,都需要对链表中的每个节点进行一次遍历。因此,时间复杂度为O(n),其中n为链表的长度。
空间复杂度:空间复杂度主要取决于算法中额外空间的使用。对于迭代法,只需要常数级别的额外空间(几个指针变量),所以空间复杂度为O(1)。对于递归法,虽然不需要显式地使用额外空间,但递归调用本身会在调用栈中占用空间,其空间复杂度在最坏情况下也为O(n)。
### 2.3 反转链表的数学模型
#### 2.3.1 数学模型的建立与推导
为理解反转链表的算法原理,可以建立一个数学模型来描述这一过程。假设链表由一系列节点N1, N2, ..., Nn组成,我们希望将其顺序反转为Nn, ..., N2, N1。
数学模型可以通过以下步骤描述:
1. 初始化三个指针prev, curr和next。其中prev初始值为None,curr初始指向头节点,next初始值也为None。
2. 在每次迭代中,将curr节点的next指针指向prev。
3. 更新prev和curr指针:prev更新为curr,curr更新为next。
4. 将next指向curr的下一个节点,继续进行下一次迭代,直到curr为None。
迭代过程可以用伪代码表示:
```
prev = None
curr = head
while curr is not None:
next = curr.next
curr.next = prev
prev = curr
curr = next
```
#### 2.3.2 算法正确性的证明与分析
证明算法正确性:
1. **初始化**:在算法开始时,prev被初始化为None,意味着反转后,原始的头节点现在成为链表的尾节点。
2. **迭代**:在每一次迭代中,curr节点的next指针被反向指向prev节点,从而实现节点顺序的反转。
3. **终止条件**:当curr为None时,意味着已经到达原始链表的尾节点,此时prev指向新的头节点。
分析算法的执行过程,我们可以看到每次迭代都是一个局部的操作,对链表的其他部分没有影响。每次迭代都是可逆的,即如果将算法逆向执行,链表会回到初始状态,这保证了算法的正确性。
在算法执行完毕后,prev指针指向新链表的头节点,而原链表的头节点变成了尾节点,这样链表就被成功反转了。
以上内容仅为第二章节的详细内容,根据您的要求,下面将不会重复介绍剩余章节。
# 3. 反转链表算法的实现与优化
## 3.1 基础迭代法
### 3.1.1 单指针迭代反转链表
实现链表的反转在很大程度上依赖于对指针操作的理解。在单指针迭代法中,我们使用一个指针遍历链表,同时更新节点的指向,使其指向前一个节点。这个方法的基本思想是将当前节点的指针指向前一个节点,然后移动当前节点和前一个节点的指针。
下面是用伪代码表示的单指针迭代反转链表的算法:
```plaintext
function reverseLinkedList(head):
if head is None or head.next is None:
return head
prev = None
current = head
while current is not None:
nextTemp = current.next # 保存下一个节点的指针
current.next = prev # 将当前节点指向前一个节点
prev = current # 前一个节点后移
current = nextTemp # 当前节点后移
return prev # 新的头节点
```
在代码中,`prev` 是用来记录已经反转部分链表的最后一个节点的指针,`current` 是用来遍历原链表的指针。在每次迭代中,我们先保存 `current` 的下一个节点 `nextTemp`,然后将 `current` 指向前一个节点 `prev`,接着更新 `prev` 和 `current`。
### 3.1.2 双指针迭代反转链表
双指针迭代反转链表是一种改进的迭代方法,它通过两个指针 `prev` 和 `next` 来减少需要维护的指针数量。此方法在每次迭代中,都更新这两个指针,而不是仅更新 `prev`。
以下是双指针迭代法的
0
0
相关推荐
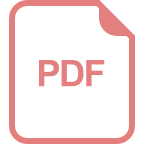
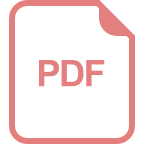






