【Java I_O与序列化】:单向链表数据持久化的高效实现
发布时间: 2024-09-11 12:43:22 阅读量: 132 订阅数: 24 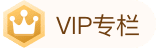
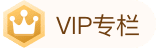
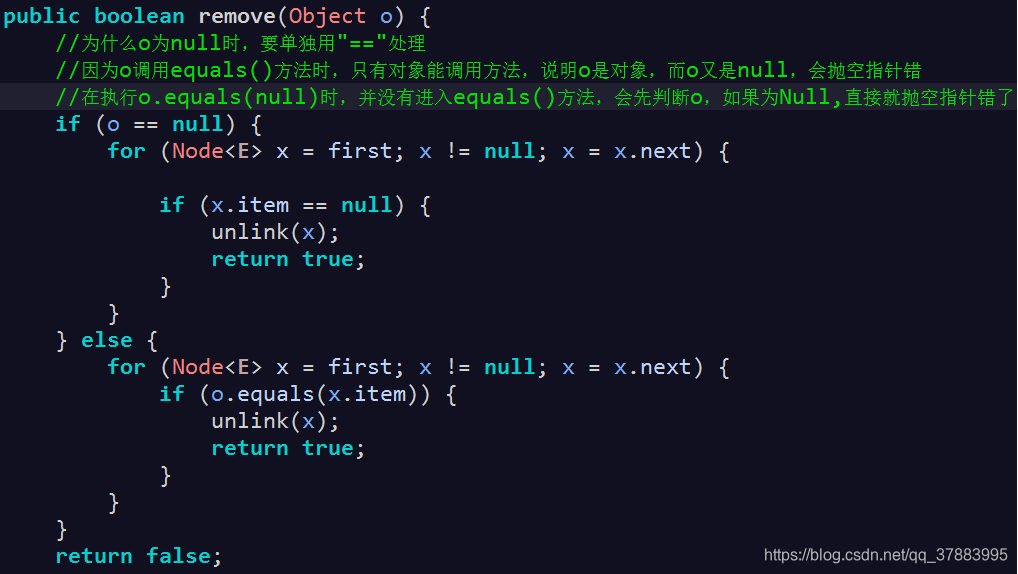
# 1. Java I/O和序列化的基础
在Java编程语言中,I/O(输入/输出)系统是一个重要的组成部分,负责与数据的输入和输出相关的所有操作。对于Java开发者来说,理解I/O和序列化的原理和实践方法是构建高效、可靠应用程序的关键。本章将简要概述Java I/O和序列化的基础知识,为后续章节关于文件系统交互、序列化机制详解、单向链表数据持久化实现、性能测试与优化等内容打下坚实基础。
## 1.1 Java I/O流概述
Java I/O流是一种以流的方式处理数据的概念,可以将其视为数据在设备之间流动的管道。输入流负责从源头(如文件、网络等)读取数据,而输出流则负责将数据写入目的地。Java中I/O流的处理是通过一系列的类和接口实现的,它们提供了丰富的方法来进行数据的读写操作,包括字节流和字符流两大类。
## 1.2 序列化的概念和重要性
序列化(Serialization)是将对象状态转换为可保持或传输的格式的过程。反序列化(Deserialization)则是序列化过程的逆过程,将格式化后的数据转换回对象。这一机制在Java中主要通过Serializable接口和相关的类实现。序列化的重要性在于它支持数据的持久化存储以及跨网络的数据传输,是构建分布式系统和网络应用中不可或缺的一部分。
# 2. Java I/O流与文件系统交互
### 2.1 Java I/O流概述
#### 2.1.1 输入流与输出流的分类
在Java中,I/O流是用来处理数据传输的抽象概念。输入流(InputStream)和输出流(OutputStream)分别用于从数据源读取数据和向数据目的地写入数据。数据源可以是文件、网络连接、内存数组等。根据处理数据的类型,Java I/O流可以分为两大类:字节流和字符流。
字节流直接以字节为单位处理数据,适用于处理二进制数据。字符流则处理字符数据,基于字符编码,因此在处理文本文件时更为方便。流中的数据可以分为有序的数据流和随机访问的数据流,分别对应于字节流的InputStream和OutputStream,以及字符流的Reader和Writer。
每种流的基类都提供了基本的读写功能。例如,InputStream类中的`read()`方法用于读取数据,而OutputStream类中的`write(int b)`方法用于写入数据。这些基类可以被派生以实现更具体的功能。
#### 2.1.2 字节流与字符流的区别和联系
字节流和字符流虽然在处理数据类型上有所区别,但它们在许多方面都有类似的操作。它们都遵循装饰者设计模式,这意味着可以通过链式调用来组合多个流实现复杂功能。
字节流的读写单位是字节,处理的是原始二进制数据,不依赖于特定的字符编码。它适用于所有类型的文件,包括文本文件。常见的字节流类包括FileInputStream、FileOutputStream、BufferedInputStream、BufferedOutputStream等。
字符流则专注于处理文本文件,其读写单位是字符。它在内部使用特定的字符编码(默认为系统默认编码)来转换字节数据。常见的字符流类包括FileReader、FileWriter、BufferedReader、BufferedWriter等。
字节流和字符流之间可以进行转换。在读取或写入文本文件时,通常会先将字节流转换为字符流,这涉及到字符编码的转换。反之,如果要将文本数据以二进制形式处理,也可以将字符流包装在字节流中。
### 2.2 文件读写操作
#### 2.2.1 使用File类进行文件操作
在Java中,File类提供了用于操作文件和目录的静态方法。尽管它不是一个流类,但它是文件系统交互的基础。File类可用于获取文件属性、创建和删除文件或目录、检查文件或目录是否存在以及列出目录内容。
以下是一些基本的使用File类操作文件的示例代码:
```java
import java.io.File;
public class FileExample {
public static void main(String[] args) {
File file = new File("example.txt");
// 检查文件是否存在
if (!file.exists()) {
try {
// 创建文件
if (file.createNewFile()) {
System.out.println("File was created!");
} else {
System.out.println("File already exists.");
}
} catch (Exception e) {
e.printStackTrace();
}
}
// 获取文件的绝对路径
System.out.println("File path: " + file.getAbsolutePath());
// 删除文件
file.delete();
}
}
```
在上述代码中,我们首先创建了一个File对象指向`example.txt`文件。然后我们使用`exists()`方法检查文件是否存在。如果文件不存在,我们使用`createNewFile()`方法来创建它。如果文件存在,我们可以使用`getAbsolutePath()`方法来获取文件的完整路径。最后,我们通过调用`delete()`方法来删除文件。
#### 2.2.2 文件通道(FileChannel)的高级操作
FileChannel提供了对文件的高级I/O操作。它只能与支持文件操作的通道关联,如FileInputStream、FileOutputStream和RandomAccessFile。FileChannel允许我们以非阻塞模式读写数据,并且可以映射文件到内存中,从而实现文件数据的直接操作。
以下是创建和使用FileChannel进行文件复制操作的示例代码:
```java
import java.io.*;
import java.nio.*;
import java.nio.channels.*;
public class FileChannelExample {
public static void main(String[] args) throws IOException {
File file1 = new File("source.txt");
File file2 = new File("destination.txt");
// 创建FileInputStream和FileOutputStream
FileInputStream fis = new FileInputStream(file1);
FileOutputStream fos = new FileOutputStream(file2);
// 获取FileChannel
FileChannel fic = fis.getChannel();
FileChannel foc = fos.getChannel();
// 创建缓冲区
ByteBuffer buffer = ByteBuffer.allocate(1024);
// 从源文件读取数据到缓冲区
while(fic.read(buffer) != -1) {
// 切换缓冲区为读模式
buffer.flip();
// 将缓冲区内容写入目标文件
while(buffer.hasRemaining()) {
foc.write(buffer);
}
// 清空缓冲区以便下次使用
buffer.clear();
}
// 关闭流和通道
fis.close();
fos.close();
}
}
```
在这段代码中,我们首先创建了两个File对象分别指向源文件和目标文件。然后,我们通过调用`getChannel()`方法从FileInputStream和FileOutputStream获取FileChannel。接着,我们创建了一个ByteBuffer作为缓冲区,用于临时存储从源文件读取的数据。通过一个循环,我们从源FileChannel读取数据到缓冲区,然后切换缓冲区为读模式,并将数据写入目标FileChannel。最后,我们清理了缓冲区并关闭了所有的流和通道。
### 2.3 文件系统的监控和管理
#### 2.3.1 文件和目录的遍历方法
在Java中,通过使用File类的list()方法或listFiles()方法,我们可以遍历文件系统中的目录和文件。list()方法返回一个字符串数组,包含目录中的文件名;而listFiles()方法返回一个File数组,每个File对象对应目录中的一个文件或子目录。
下面是一个遍历目录并打印出所有文件和子目录名称的示例代码:
```java
import java.io.File;
public class DirectoryTraversalExample {
public static void main(String[] args) {
File directory = new File("path/to/directory");
// 递归遍历目录
traverseDirectory(directory);
}
public static void traverseDirectory(File dir) {
File[] files = dir.listFiles();
if (files != null) {
for (File *** {
if (file.isDirectory()) {
System.out.println("Directory: " + file.getPath());
traverseDirectory(file); // 递归调用
} else {
System.out.println("File: " + file.getPath());
}
}
}
}
}
```
在这个例子中,我们定义了一个`traverseDirectory`方法来递归遍历目录。我们检查每个File对象是否为目录,如果是,则递归地调用自身;如果是文件,则直接打印文件路径。
#### 2.3.2 文件属性的获取与修改
Java的File类提供了方法来获取和修改文件的属性,比如文件的大小、最后修改时间、是否是隐藏文件等。以下是如何使用File类获取文件属性的代码示例:
```java
import java.io.File;
import java.util.Date;
public class FileAttributeExample {
public static void main(String[] args) {
File file = new File("example.txt");
// 检查文件是否存在
if (file.exists()) {
// 获取文件大小
long lengt
```
0
0
相关推荐
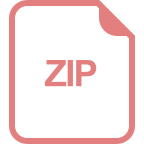
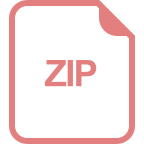
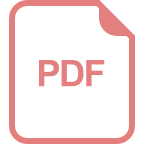





