给出实现上面问题的python代码
时间: 2024-12-10 22:38:05 浏览: 5
为了实现上述遗传算法路径规划的问题,我们可以采用Python编写一个简单版本。首先,需要导入必要的库并定义基本的类和函数。这里是一个简化的例子,假设我们使用`numpy`处理矩阵,以及`random`模块生成随机路径和进行选择、交叉、变异等操作:
```python
import numpy as np
import random
# 定义网格地图类
class GridMap:
def __init__(self, width, height, obstacles):
self.map = np.zeros((width, height), dtype=int)
for x, y in obstacles:
self.map[x][y] = -1 # 障碍位置设为负数
# 遗传算法核心类
class GeneticAlgorithm:
def __init__(self, map_data, start, end, pop_size=100, mutation_rate=0.01):
self.grid_map = GridMap(*map_data)
self.start, self.end = start, end
self.pop_size, self.mutation_rate = pop_size, mutation_rate
self.population = self.create_population()
def create_population(self):
paths = []
for _ in range(self.pop_size):
path = [self.start]
current = self.start
while not self.is_goal(current):
possible_neighbors = self.get_neighbors(current)
if possible_neighbors:
next_step = random.choice(possible_neighbors)
else:
break
path.append(next_step)
current = next_step
paths.append(path)
return paths
def fitness_function(self, path):
return sum([self.grid_map.map[path[i]][path[i+1]] for i in range(len(path)-1)])
# ...继续添加选择、交叉和变异的方法...
# 辅助方法
def is_goal(self, position):
return position == self.end and all(self.grid_map.map[position])
def get_neighbors(self, position):
valid_neighbors = [(position[0]+dx, position[1]+dy) for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)] if self.grid_map.map[position[0]+dx][position[1]+dy] >= 0]
return valid_neighbors
# 示例使用
ga = GeneticAlgorithm((10, 10), (0, 0), (9, 9)) # 假设10x10的地图,起点(0,0),终点(9,9)
best_path = ga.run() # 调用算法找出最佳路径
```
这只是一个基本框架,实际应用中可能还需要完善循环终止条件、优化算法细节等。
阅读全文
相关推荐
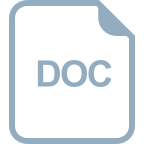
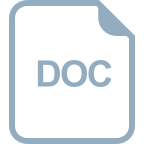
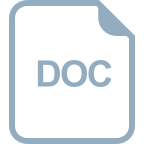




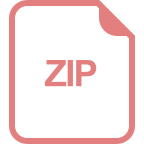










