用c++写一段简单的词法分析代码
时间: 2024-04-21 10:04:27 浏览: 276
下面是一个简单的 C++ 词法分析器示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义符号类型
enum TokenType {
INTEGER, PLUS, MINUS, MUL, DIV, LPAREN, RPAREN, END
};
// 定义符号结构体
struct Token {
TokenType type;
int value;
};
// 定义词法分析器类
class Lexer {
public:
Lexer(string text) : text(text), pos(0), current_char(text[0]) {}
// 解析下一个符号
Token get_next_token() {
while (current_char != '\0') {
if (isdigit(current_char)) {
return Token{INTEGER, integer()};
} else if (current_char == '+') {
advance();
return Token{PLUS, 0};
} else if (current_char == '-') {
advance();
return Token{MINUS, 0};
} else if (current_char == '*') {
advance();
return Token{MUL, 0};
} else if (current_char == '/') {
advance();
return Token{DIV, 0};
} else if (current_char == '(') {
advance();
return Token{LPAREN, 0};
} else if (current_char == ')') {
advance();
return Token{RPAREN, 0};
} else {
error();
}
}
return Token{END, 0};
}
private:
string text; // 待解析的文本
int pos; // 当前位置
char current_char; // 当前字符
// 将 pos 移动一位,并更新当前字符
void advance() {
pos++;
if (pos >= text.size()) {
current_char = '\0';
} else {
current_char = text[pos];
}
}
// 解析整数
int integer() {
string result = "";
while (isdigit(current_char)) {
result += current_char;
advance();
}
return stoi(result);
}
// 报错
void error() {
cout << "Error parsing input" << endl;
exit(1);
}
};
int main() {
string text = "3+5*2/(1-5)";
Lexer lexer(text);
Token token = lexer.get_next_token();
while (token.type != END) {
cout << "{ type: " << token.type << ", value: " << token.value << " }" << endl;
token = lexer.get_next_token();
}
cout << "{ type: " << token.type << ", value: " << token.value << " }" << endl;
return 0;
}
```
使用示例:
输入:`3+5*2/(1-5)`。
输出:
```
{ type: INTEGER, value: 3 }
{ type: PLUS, value: 0 }
{ type: INTEGER, value: 5 }
{ type: MUL, value: 0 }
{ type: INTEGER, value: 2 }
{ type: DIV, value: 0 }
{ type: LPAREN, value: 0 }
{ type: INTEGER, value: 1 }
{ type: MINUS, value: 0 }
{ type: INTEGER, value: 5 }
{ type: RPAREN, value: 0 }
{ type: END, value: 0 }
```
该词法分析器只支持解析加、减、乘、除、括号和整数。在解析过程中,先判断当前字符的类型,然后根据不同类型的字符返回不同类型的 Token。如果遇到无法识别的字符,则报错。
阅读全文
相关推荐



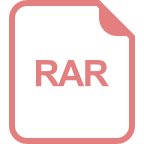

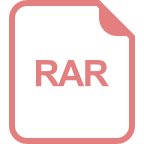
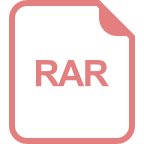
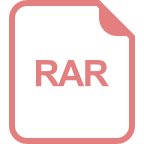
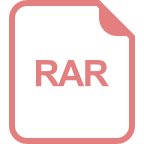
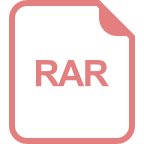
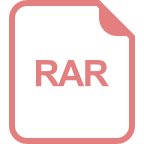





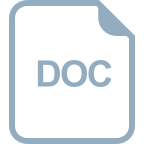