给我一段使用c++的词法分析器代码
时间: 2024-01-12 11:05:51 浏览: 20
以下是一个简单的使用 C 语言实现的词法分析器的代码示例:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAX_TOKEN_LEN 100
typedef enum {
INT, FLOAT, IDENTIFIER, OPERATOR, DELIMITER, KEYWORD
} TokenType;
typedef struct {
char token[MAX_TOKEN_LEN];
TokenType tokenType;
} Token;
int isOperator(char c) {
char operators[] = "+-*/=";
int i;
for (i = 0; i < strlen(operators); i++) {
if (c == operators[i]) {
return 1;
}
}
return 0;
}
int isDelimiter(char c) {
char delimiters[] = "();,{}[]";
int i;
for (i = 0; i < strlen(delimiters); i++) {
if (c == delimiters[i]) {
return 1;
}
}
return 0;
}
int isKeyword(char* token) {
char keywords[][10] = {"int", "float", "if", "else", "while", "do", "for"};
int i;
for (i = 0; i < sizeof(keywords) / sizeof(keywords[0]); i++) {
if (strcmp(token, keywords[i]) == 0) {
return 1;
}
}
return 0;
}
Token getNextToken(char* code) {
Token token;
int i = 0;
while (isspace(code[i])) {
i++;
}
if (isalpha(code[i])) {
while (isalnum(code[i]) || code[i] == '_') {
token.token[i] = code[i];
i++;
}
token.token[i] = '\0';
if (isKeyword(token.token)) {
token.tokenType = KEYWORD;
} else {
token.tokenType = IDENTIFIER;
}
} else if (isdigit(code[i])) {
while (isdigit(code[i])) {
token.token[i] = code[i];
i++;
}
if (code[i] == '.') {
i++;
while (isdigit(code[i])) {
token.token[i] = code[i];
i++;
}
token.tokenType = FLOAT;
} else {
token.tokenType = INT;
}
} else if (isOperator(code[i])) {
token.token[0] = code[i];
token.tokenType = OPERATOR;
i++;
} else if (isDelimiter(code[i])) {
token.token[0] = code[i];
token.tokenType = DELIMITER;
i++;
} else {
token.token[0] = '\0';
token.tokenType = -1;
i++;
}
return token;
}
int main() {
char code[] = "int a = 10; float b = 3.14; if (a > b) { printf(\"a is greater than b\"); }";
Token token;
do {
token = getNextToken(code);
printf("%s\t%d\n", token.token, token.tokenType);
code += strlen(token.token);
} while (strlen(token.token) > 0);
return 0;
}
```
这个词法分析器可以处理整型、浮点型、标识符、运算符、分隔符和关键字等各种类型的词法单元。在 `getNextToken` 函数中,通过判断输入代码的第一个字符来确定当前词法单元的类型,并根据类型解析出词法单元的内容。最后,将解析出的词法单元存储到一个 `Token` 结构体中并返回。在主函数中,循环调用 `getNextToken` 函数来逐个输出解析出的词法单元及其类型。
相关推荐
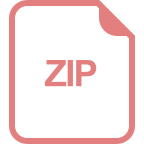
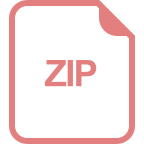
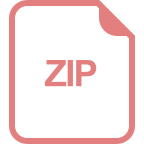















