pl/0语言词法分析器C++
时间: 2023-05-24 14:02:58 浏览: 186
A:您好,我是ChitGPT语言模型,很高兴回答您的问题。
pl/0语言是一种过程性语言,词法分析器C的作用是将用户输入的源代码转化为一个个单词或符号,并建立对应的单词符号表。在编译器的编译过程中,词法分析是其重要的一个阶段。
下面是一个简单的pl/0语言词法分析器C示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAXLEN 100 // 单词最大长度
char token[MAXLEN+1]; // 保存当前单词的数组
int tokenType; // 当前单词的类型
// 判断是否关键字
int isKeyword(char *word) {
char *keywords[] = {"const", "var", "procedure", "begin", "end", "if", "then",
"while", "do", "call", "odd"};
for(int i=0; i<11; i++) {
if(strcmp(word, keywords[i]) == 0) return i+1;
}
return 0;
}
// 获取下一个字符
int getNextChar() {
static int index = 0;
static char line[MAXLEN+1];
int i;
if(index == 0) {
if(fgets(line, MAXLEN+1, stdin) == NULL) return EOF;
}
if((i = line[index++]) == '\0') return EOF;
return i;
}
// 获取下一个单词
void getNextToken() {
int i = 0, ch;
while(isspace(ch = getNextChar()));
if(isalpha(ch)) {
while(isalpha(ch) || isdigit(ch)) {
token[i++] = ch;
ch = getNextChar();
}
token[i] = '\0';
tokenType = isKeyword(token);
if(tokenType == 0) tokenType = 11;
index--;
} else if(isdigit(ch)) {
while(isdigit(ch)) {
token[i++] = ch;
ch = getNextChar();
}
token[i] = '\0';
tokenType = 10;
index--;
} else {
switch(ch) {
case '(':
token[0] = '(';
token[1] = '\0';
tokenType = 21; break;
case ')':
token[0] = ')';
token[1] = '\0';
tokenType = 22; break;
case '+':
token[0] = '+';
token[1] = '\0';
tokenType = 23; break;
case '-':
token[0] = '-';
token[1] = '\0';
tokenType = 24; break;
case '*':
token[0] = '*';
token[1] = '\0';
tokenType = 25; break;
case '/':
token[0] = '/';
token[1] = '\0';
tokenType = 26; break;
case '=':
token[0] = '=';
token[1] = '\0';
tokenType = 27; break;
case ',':
token[0] = ',';
token[1] = '\0';
tokenType = 28; break;
case '.':
token[0] = '.';
token[1] = '\0';
tokenType = 29; break;
case ':':
if((ch = getNextChar()) == '=') {
token[0] = ':';
token[1] = '=';
token[2] = '\0';
tokenType = 20;
} else {
printf("Lexical Error: Wrong Character '%c'.\n", ch);
exit(1);
}
break;
default:
printf("Lexical Error: Wrong Character '%c'.\n", ch);
exit(1);
}
}
}
int main() {
while(tokenType != 29) {
getNextToken();
printf("token = %s, tokenType = %d\n", token, tokenType);
memset(token, 0, sizeof(token));
}
return 0;
}
```
以上是一个pl/0语言词法分析器C代码示例,主要利用C语言的库函数实现了对输入源代码的词法分析过程,并输出每个单词的类型及内容。在实际编译器中,还需添加符号表的建立、错误处理等相关功能。
阅读全文
相关推荐
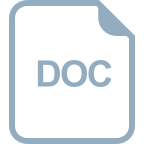
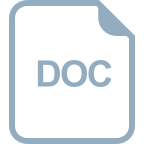
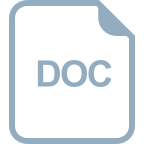
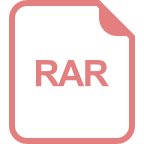

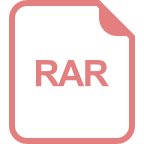
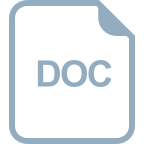
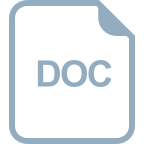
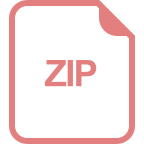
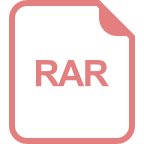
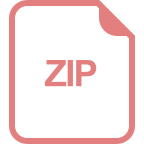
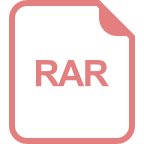
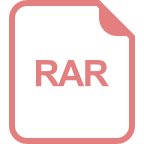
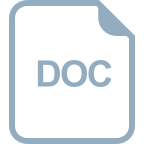
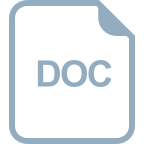


