array2d<rgb_pixel> 转 cv::mat
时间: 2024-01-20 07:02:57 浏览: 23
你可以使用 OpenCV 库中的 `cv::Mat` 类来实现 `array2d<rgb_pixel>` 到 `cv::Mat` 的转换。下面是一个简单的示例代码:
```c++
// 假设有一个名为 img 的 array2d<rgb_pixel> 对象
cv::Mat mat(img.nr(), img.nc(), CV_8UC3);
for (int r = 0; r < img.nr(); ++r) {
for (int c = 0; c < img.nc(); ++c) {
const dlib::rgb_pixel& p = img[r][c];
cv::Vec3b& q = mat.at<cv::Vec3b>(r, c);
q[0] = p.blue;
q[1] = p.green;
q[2] = p.red;
}
}
```
这里我们先创建了一个 `cv::Mat` 对象,并设置其大小为 `img.nr()` 行、`img.nc()` 列,像素格式为 8 位 3 通道。然后我们使用双重循环遍历 `img` 中的每个像素,并将其转换为 `cv::Vec3b` 类型的像素值,再将其赋值给对应位置的 `mat` 中的像素。注意到 `cv::Mat` 中的像素格式是 BGR,而 `dlib::rgb_pixel` 中的像素格式是 RGB,因此需要做一些通道的交换。
相关问题
array2d<rgb_pixel> 转cv::mat 后mat是empty
在将 `array2d<rgb_pixel>` 转换为 `cv::Mat` 时,需要注意两个问题:
1. `array2d<rgb_pixel>` 的存储顺序是按行存储的,而 `cv::Mat` 的存储顺序是按列存储的,因此需要进行转置操作。
2. `array2d<rgb_pixel>` 的像素值的类型是 `rgb_pixel`,而 `cv::Mat` 的像素值的类型是 `cv::Vec3b`,需要进行类型转换。
下面是一个示例代码,可以将 `array2d<rgb_pixel>` 转换为 `cv::Mat`:
```cpp
#include <iostream>
#include <dlib/image_processing.h>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace dlib;
int main()
{
// 创建一个 array2d<rgb_pixel>
array2d<rgb_pixel> img(100, 100);
for (int r = 0; r < img.nr(); r++) {
for (int c = 0; c < img.nc(); c++) {
img[r][c] = rgb_pixel(r, c, 255);
}
}
// 将 array2d<rgb_pixel> 转换为 cv::Mat
cv::Mat mat(img.nc(), img.nr(), CV_8UC3, cv::Scalar(0, 0, 0));
for (int r = 0; r < img.nr(); r++) {
for (int c = 0; c < img.nc(); c++) {
cv::Vec3b& pixel = mat.at<cv::Vec3b>(c, r);
rgb_pixel& dlib_pixel = img[r][c];
pixel[0] = dlib_pixel.blue;
pixel[1] = dlib_pixel.green;
pixel[2] = dlib_pixel.red;
}
}
cv::transpose(mat, mat); // 转置
// 显示结果
cv::imshow("image", mat);
cv::waitKey(0);
return 0;
}
```
在这个示例代码中,我使用了一个简单的方法来将 `array2d<rgb_pixel>` 转换为 `cv::Mat`,也就是先创建一个空的 `cv::Mat`,然后逐个像素进行复制和类型转换。最后再使用 `cv::transpose` 将 `cv::Mat` 转置,以满足存储顺序的要求。
如果你还是遇到了 `cv::Mat` 是空的问题,可以检查一下你的 `array2d<rgb_pixel>` 是否为空,以及是否正确地转换为了 `cv::Mat`。
c++ 将cv::mat 转为 array2d<rgb_pixel>
你可以使用以下代码将 cv::Mat 转换为 dlib 的 array2d<rgb_pixel> 类型:
```cpp
#include <dlib/image_processing.h>
#include <dlib/image_io.h>
using namespace dlib;
// 将 cv::Mat 转为 array2d<rgb_pixel>
array2d<rgb_pixel> mat_to_array2d(const cv::Mat& img)
{
// 创建一个空的 array2d<rgb_pixel> 对象
array2d<rgb_pixel> out_img(img.rows, img.cols);
// 遍历每一个像素,将其赋值给 array2d<rgb_pixel> 对象
for (int r = 0; r < img.rows; ++r) {
for (int c = 0; c < img.cols; ++c) {
const cv::Vec3b& bgr = img.at<cv::Vec3b>(r, c);
out_img[r][c] = rgb_pixel(bgr[2], bgr[1], bgr[0]);
}
}
return out_img;
}
```
然后你就可以使用以上函数将 cv::Mat 转换为 dlib 的 array2d<rgb_pixel> 类型了。
相关推荐
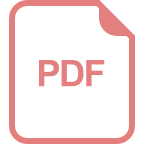
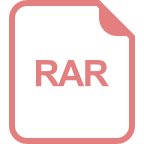













