试编写java代码,实现复数求和运算,要求能够满足两个复数数、复数数组的求和运算需求
时间: 2023-06-20 07:04:26 浏览: 95
以下是实现复数求和运算的Java代码:
```java
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
double newReal = this.real + other.real;
double newImag = this.imag + other.imag;
return new Complex(newReal, newImag);
}
public static Complex add(Complex[] complexArray) {
Complex sum = new Complex(0, 0);
for (Complex c : complexArray) {
sum = sum.add(c);
}
return sum;
}
@Override
public String toString() {
return real + " + " + imag + "i";
}
}
```
这个类包含了两个方法:
- `add(Complex other)`:实现两个复数相加的操作。
- `add(Complex[] complexArray)`:实现多个复数相加的操作。
使用示例如下:
```java
public class Main {
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex c3 = new Complex(5, 6);
Complex[] complexArray = { c1, c2, c3 };
Complex sum = Complex.add(complexArray);
System.out.println("Sum: " + sum);
}
}
```
输出结果为:
```
Sum: 9.0 + 12.0i
```
阅读全文
相关推荐

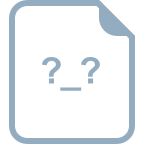
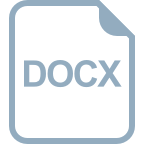
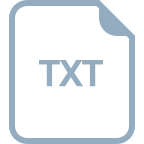
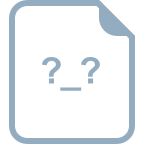
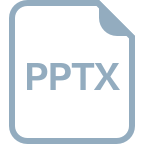
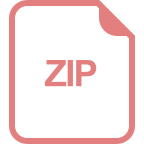
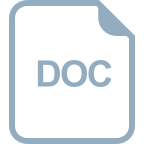
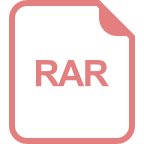
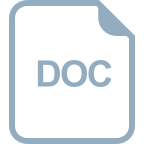
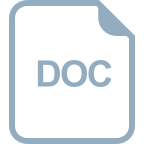
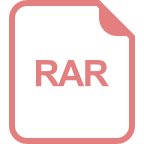
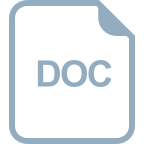
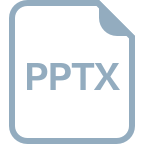