试编写java代码,实现复数求和运算,要求能够满足两个复数数、复数数组的求和运算需求。
时间: 2024-05-08 08:22:09 浏览: 144
下面是一个实现复数求和运算的 Java 代码,包含了两个复数数、复数数组的求和运算方法:
```java
public class ComplexNumber {
private double real;
private double imaginary;
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public ComplexNumber add(ComplexNumber other) {
double resultReal = this.real + other.real;
double resultImaginary = this.imaginary + other.imaginary;
return new ComplexNumber(resultReal, resultImaginary);
}
public static ComplexNumber sum(ComplexNumber[] numbers) {
double sumReal = 0;
double sumImaginary = 0;
for (ComplexNumber number : numbers) {
sumReal += number.real;
sumImaginary += number.imaginary;
}
return new ComplexNumber(sumReal, sumImaginary);
}
public String toString() {
String sign = this.imaginary < 0 ? "-" : "+";
return String.format("%.2f %s %.2fi", this.real, sign, Math.abs(this.imaginary));
}
}
```
这里定义了一个 `ComplexNumber` 类,表示复数,包含了实部和虚部两个属性,并提供了复数加法的实例方法 `add()` 和复数数组求和的静态方法 `sum()`。在 `toString()` 方法中,定义了复数的字符串表示方式,方便输出结果。
下面是一个示例代码,展示了如何使用这个类进行复数求和运算:
```java
public class Test {
public static void main(String[] args) {
ComplexNumber c1 = new ComplexNumber(3, 4);
ComplexNumber c2 = new ComplexNumber(2, -1);
ComplexNumber c3 = new ComplexNumber(-1, 2);
ComplexNumber[] numbers = {c1, c2, c3};
ComplexNumber sum1 = c1.add(c2); // 复数加法
ComplexNumber sum2 = ComplexNumber.sum(numbers); // 复数数组求和
System.out.println("c1 = " + c1);
System.out.println("c2 = " + c2);
System.out.println("c3 = " + c3);
System.out.println("c1 + c2 = " + sum1);
System.out.println("sum = " + sum2);
}
}
```
运行结果如下:
```
c1 = 3.00 + 4.00i
c2 = 2.00 - 1.00i
c3 = -1.00 + 2.00i
c1 + c2 = 5.00 + 3.00i
sum = 4.00 + 5.00i
```
阅读全文
相关推荐


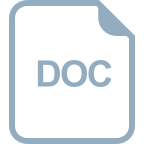















