C++设计一个可进行复数运算的演示程序。 实现下列六种基本运算: (1)由输入的实部和虚部生成一个复数; (2)两个复数求和; (3)两个复数求差; (4)两个复数求积; (5)从已知复数中分离出实部; (6)从已知复数中分离出虚部。运算结果以相应的复数或实数的表示形式显示。
时间: 2024-10-13 07:05:38 浏览: 43
为了创建一个简单的C++程序来处理复数运算,我们可以使用结构体定义复数,并提供必要的成员函数来实现上述六个操作。以下是实现这个功能的一个示例:
```cpp
#include <iostream>
using namespace std;
// 定义复数结构体
struct Complex {
double real; // 实部
double imag; // 虚部
// 构造函数,用于初始化复数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 运算符重载
Complex operator+(const Complex& other) const {
return {real + other.real, imag + other.imag};
}
Complex operator-(const Complex& other) const {
return {real - other.real, imag - other.imag};
}
Complex operator*(const Complex& other) const {
double newReal = real * other.real - imag * other.imag;
double newImag = real * other.imag + imag * other.real;
return {newReal, newImag};
}
Complex conjugate() const {
return {real, -imag}; // 求共轭
}
void display() const {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
// (1) 输入复数
Complex c1, c2;
cout << "Enter the real and imaginary parts of complex number 1: ";
cin >> c1.real >> c1.imag;
cout << "Enter the real and imaginary parts of complex number 2: ";
cin >> c2.real >> c2.imag;
// (2) 加法
Complex sum = c1 + c2;
cout << "Sum: ";
sum.display();
// (3) 减法
Complex diff = c1 - c2;
cout << "Difference: ";
diff.display();
// (4) 积
Complex prod = c1 * c2;
cout << "Product: ";
prod.display();
// (5) 分离实部
cout << "Real part of first complex number: " << c1.real << endl;
// (6) 分离虚部
cout << "Imaginary part of second complex number: " << c2.imag << endl;
return 0;
}
```
运行此程序,用户可以输入两个复数并查看它们之间的加、减、乘以及各自的实部和虚部。当你复制并运行这个代码片段时,别忘了添加适当的错误检查和异常处理,特别是在用户输入非数字值时。
阅读全文
相关推荐
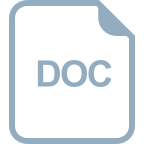
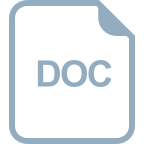
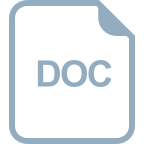
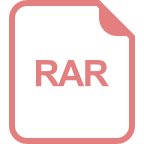
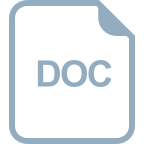












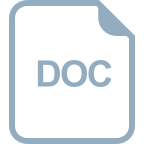
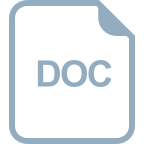