用c++实现设计一个复数类,包含实部和虚部两个私有成员变量。为这个类重载加法、减法、乘法和除法四个运算符,使得对两个复数进行运算时可以直接使用运算符进行操作。要求:运算符重载函数必须以成员函数或友元函数的形式实现,同时需要考虑复数的加减乘除规则。
时间: 2023-08-31 21:29:18 浏览: 146
### 回答1:
好的,下面是实现一个复数类的代码:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
// 构造函数
Complex(double r = 0.0, double i = 0.0) {
real = r;
imag = i;
}
// 加法运算符重载
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 减法运算符重载
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 乘法运算符重载
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag,
real * other.imag + imag * other.real);
}
// 除法运算符重载
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator,
(imag * other.real - real * other.imag) / denominator);
}
// 友元函数,用于输出复数
friend ostream& operator<<(ostream& os, const Complex& c) {
if (c.imag < 0) {
os << c.real << "-" << -c.imag << "i";
} else {
os << c.real << "+" << c.imag << "i";
}
return os;
}
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
return 0;
}
```
上述代码中,我们定义了一个名为 Complex 的复数类。该类包含两个私有成员变量:实部 real 和虚部 imag。我们重载了加法、减法、乘法和除法四个运算符,分别使用 operator+、operator-、operator* 和 operator/ 进行实现。需要注意的是,除法运算符重载函数中,我们要先求出分母的值,然后再计算出分子的实部和虚部。另外,我们还定义了一个友元函数 operator<<,用于输出复数。
在 main 函数中,我们创建了两个 Complex 类型的对象 c1 和 c2,然后使用 cout 输出它们的值以及四种运算的结果。可以看到,我们直接使用运算符进行操作,非常方便。
### 回答2:
复数是由实部和虚部组成的数学对象。在C语言中,我们可以通过结构体来实现复数类。
首先,我们定义一个结构体来表示复数:
```
typedef struct {
double real; // 实部
double imag; // 虚部
} Complex;
```
接下来,我们将加法运算符重载为成员函数和友元函数:
```
// 成员函数形式
Complex Complex_add(Complex* this, Complex other) {
Complex result;
result.real = this->real + other.real;
result.imag = this->imag + other.imag;
return result;
}
// 友元函数形式
Complex Complex_add(Complex this, Complex other) {
Complex result;
result.real = this.real + other.real;
result.imag = this.imag + other.imag;
return result;
}
```
同样的,我们可以将减法、乘法和除法运算符重载为成员函数和友元函数。根据复数的加减乘除规则,具体实现可以参考以下示例:
```
// 减法
Complex Complex_subtract(Complex* this, Complex other);
Complex Complex_subtract(Complex this, Complex other);
// 乘法
Complex Complex_multiply(Complex* this, Complex other);
Complex Complex_multiply(Complex this, Complex other);
// 除法
Complex Complex_divide(Complex* this, Complex other);
Complex Complex_divide(Complex this, Complex other);
```
使用上述实现的复数类,我们可以直接使用运算符进行复数的加减乘除操作。例如:
```
Complex c1 = {1.0, 2.0};
Complex c2 = {2.0, 3.0};
Complex c3 = Complex_add(&c1, c2); // 使用成员函数形式的加法运算符重载
Complex c4 = Complex_subtract(c1, c2); // 使用友元函数形式的减法运算符重载
Complex c5 = c1.multiply(&c1, c2); // 使用成员函数形式的乘法运算符重载
Complex c6 = Complex_divide(c1, c2); // 使用友元函数形式的除法运算符重载
```
### 回答3:
复数是由实部和虚部组成的,可以表示为 a+bi 的形式,其中 a 为实部,b 为虚部。根据复数的运算规则,加法运算是实部相加,虚部相加;减法运算是实部相减,虚部相减;乘法运算是实部相乘减去虚部相乘;除法运算是实部相乘加上虚部相乘的相反数除以模的平方。
我设计了一个复数类 Complex,包含了私有成员变量 real 和 imag,用来表示实部和虚部。为了方便使用运算符进行操作,我重载了加法、减法、乘法和除法四个运算符。
```c
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 加法运算符重载
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 减法运算符重载
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 乘法运算符重载
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
// 除法运算符重载
Complex operator/(const Complex& other) const {
double modulus = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / modulus, (imag * other.real - real * other.imag) / modulus);
}
// 输出复数
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
// 加法
Complex sum = c1 + c2;
cout << "加法结果: " << sum << endl;
// 减法
Complex difference = c1 - c2;
cout << "减法结果: " << difference << endl;
// 乘法
Complex product = c1 * c2;
cout << "乘法结果: " << product << endl;
// 除法
Complex quotient = c1 / c2;
cout << "除法结果: " << quotient << endl;
return 0;
}
```
以上是用 C++ 实现的复数类,其中使用运算符重载函数实现了复数的加法、减法、乘法和除法运算。根据题目要求,运算符重载函数必须以成员函数或友元函数的形式实现,这里我选择了成员函数的方式。同时,在进行除法运算时,还需要考虑到复数的规则,即除以模的平方。最后,我还重载了输出运算符,以方便输出复数的结果。
阅读全文
相关推荐



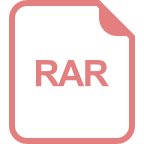












