设计一个复数Complex类,用友元函数重载乘法运算符(*),用成员函数重载除法运算符(/),完成两个复数对象的乘除加减法,使用c++编写
时间: 2024-03-02 07:49:47 浏览: 18
好的,我可以为您编写一个Complex类,实现乘除加减法的重载运算符。代码如下:
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
friend Complex operator+(const Complex &c1, const Complex &c2); // 友元函数,重载加法运算符
friend Complex operator-(const Complex &c1, const Complex &c2); // 友元函数,重载减法运算符
friend Complex operator*(const Complex &c1, const Complex &c2); // 友元函数,重载乘法运算符
Complex operator/(const Complex &rhs) const; // 重载除法运算符
void display() const;
private:
double real; // 实部
double imag; // 虚部
};
Complex operator+(const Complex &c1, const Complex &c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
Complex operator-(const Complex &c1, const Complex &c2) {
return Complex(c1.real - c2.real, c1.imag - c2.imag);
}
Complex operator*(const Complex &c1, const Complex &c2) {
double real = c1.real * c2.real - c1.imag * c2.imag;
double imag = c1.real * c2.imag + c1.imag * c2.real;
return Complex(real, imag);
}
Complex Complex::operator/(const Complex &rhs) const {
double r = rhs.real * rhs.real + rhs.imag * rhs.imag;
return Complex((real * rhs.real + imag * rhs.imag) / r, (imag * rhs.real - real * rhs.imag) / r);
}
void Complex::display() const {
cout << "(" << real << ", " << imag << "i)" << endl;
}
int main() {
Complex c1(2, 3);
Complex c2(4, 5);
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
Complex c5 = c1 * c2;
Complex c6 = c1 / c2;
c1.display();
c2.display();
c3.display();
c4.display();
c5.display();
c6.display();
return 0;
}
```
在上面的代码中,我们定义了一个Complex类,其中包括实部和虚部两个成员变量。我们重载了加法、减法、乘法和除法运算符,分别用于实现两个复数对象的加、减、乘、除运算。在加法、减法和乘法运算符的重载函数中,我们使用了友元函数的方式进行重载,而在除法运算符的重载函数中,我们使用了成员函数的方式进行重载。在主函数中,我们创建了两个复数对象c1和c2,并对它们进行了加、减、乘、除运算,将结果保存在c3、c4、c5和c6中,并输出了所有的复数对象。
需要注意的是,在加法、减法和乘法运算符的重载函数中,我们直接访问了Complex类的私有成员变量real和imag,因此需要将这些函数声明为Complex类的友元函数。
相关推荐
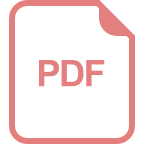
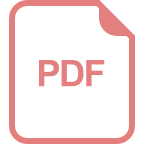
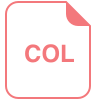
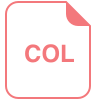
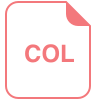
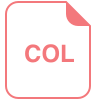






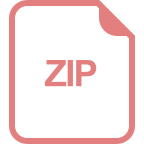