using System; using System.Windows.Forms; using System.Xml; namespace fruit { public partial class Form1 : Form { public XmlDocument doc; public XmlElement root; public Form1() { InitializeComponent(); } private void button2_Click(object sender, EventArgs e) { doc = new XmlDocument(); XmlDeclaration declare = doc.CreateXmlDeclaration("1.0", "utf-8", "yes"); doc.AppendChild(declare); root = doc.CreateElement("水果列表"); doc.AppendChild(root); XmlElement list = doc.CreateElement("水果"); XmlAttribute name = doc.CreateAttribute("名称"); name.Value = textBox1.Text; list.Attributes.Append(name); XmlElement prize = doc.CreateElement("价格"); XmlText prize1 = doc.CreateTextNode(textBox2.Text); prize.AppendChild(prize1); XmlElement num = doc.CreateElement("数量"); XmlText num1 = doc.CreateTextNode(textBox3.Text); num.AppendChild(num1); root.AppendChild(list); list.AppendChild(prize); list.AppendChild(num); } private void button3_Click(object sender, EventArgs e) { if (saveFileDialog1.ShowDialog() == DialogResult.OK) { string fruit = saveFileDialog1.FileName; doc.Save(fruit); MessageBox.Show("保存成功"); textBox1.Text = ""; textBox2.Text = ""; textBox3.Text = ""; } } private void Form1_Load(object sender, EventArgs e) { } } }是什么意思
时间: 2024-02-10 21:33:06 浏览: 135
这是一个 C# 的 Windows 窗体应用程序,用于创建和保存一个简单的 XML 文件。当用户在文本框中输入水果名称、价格和数量后,点击“添加”按钮,程序会创建一个名为“水果列表”的根元素,然后将用户输入的水果信息作为子元素添加到根元素下。用户可以点击“保存”按钮将生成的 XML 文件保存到本地磁盘上。
相关问题
将上述程序填写到下面的程序里using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Reflection; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace WindowsFormsApp1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { } } }
好的,将上述C# Switch程序填写到Windows窗体应用程序中的button1_Click事件中,可以这样写:
```csharp
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
Console.WriteLine("请选择一个数字(1~3):");
int num = int.Parse(Console.ReadLine());
switch (num)
{
case 1:
MessageBox.Show("您选择了数字1。");
break;
case 2:
MessageBox.Show("您选择了数字2。");
break;
case 3:
MessageBox.Show("您选择了数字3。");
break;
default:
MessageBox.Show("您输入的数字不在范围内。");
break;
}
}
}
}
```
这个程序会在窗体中添加一个按钮,当用户点击该按钮时,会弹出一个提示框,要求用户输入一个数字。然后使用switch语句根据用户的选择输出相应的信息。如果用户输入的数字不在1~3的范围内,则输出一个默认的提示信息。
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; //添加ABB库引用 using ABB.Robotics.Controllers; using ABB.Robotics.Controllers.Discovery; using ABB.Robotics; namespace RobotManager2 { public partial class Form1 : Form { //扫描器 private NetworkScanner scanner=null; //控制器集合 private ControllerInfoCollection controllers=null; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { } void ScanConroller() { //创建扫描器 this.scanner = new NetworkScanner(); //启动扫描 this.scanner.Scan(); } } }
这段代码是一个 C# 的 Windows Forms 应用程序,其中使用了 ABB 公司的机器人控制器库。主要实现了以下功能:
1. 创建了一个窗体 Form1;
2. 实现了窗体加载事件 Form1_Load;
3. 创建了一个 NetworkScanner 对象 scanner,用于扫描网络中可用的机器人控制器;
4. 创建了一个 ControllerInfoCollection 对象 controllers,用于存储扫描到的控制器信息。
在 Form1_Load 事件中,可以调用 ScanConroller 方法来启动扫描器,获取可用的机器人控制器列表。此处只是代码的一部分,如果需要使用 ABB 机器人控制器库进行开发,还需要补充其他代码来实现具体的功能。
阅读全文
相关推荐
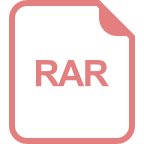
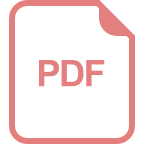


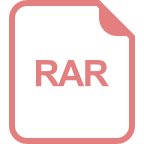
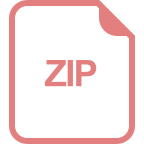
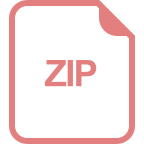
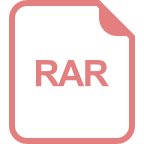
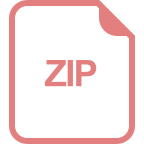
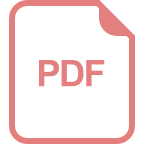
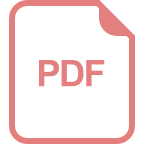
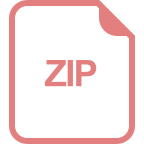
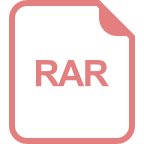
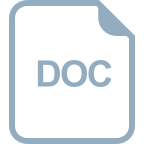
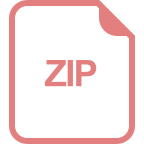