用c++写代码输入一系列复数的编号、实部、虚部,按以下要求进行排序。 排序要求为:先按复数“模的整数部分”从小到大排序,如果“模的整数部分”相同,则按实部从小到大排,如果实部又相同,则按虚部从小到大排。 打印排序后的复数编号。 输入 前面若干行,分别代表若干个复数,每行3个整数a,b,p,分别代表复数编号、实部、虚部,其中:1<=a<=2000,1<=b,p<=100。 最后一行,输入0,表示结束。 输出 输出1行,若干个数字,为按要求排序之后的复数编号。 样例输入 Copy 1 2 5 2 3 3 3 4 9 4 8 7 5 8 11 6 6 9 7 4 10 8 9 3 9 9 2 10 8 6 11 2 13 0 样例输出 Copy 2 1 3 9 8 7 6 10 4 11 5
时间: 2024-04-14 13:28:50 浏览: 134
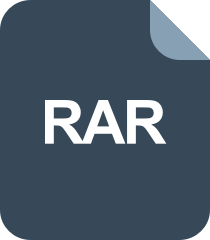
C++复数类实部和虚部
以下是使用C++编写的代码,实现了按要求对复数进行排序:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
struct ComplexNumber {
int id;
int real;
int imaginary;
int modInteger;
ComplexNumber(int id, int real, int imaginary) {
this->id = id;
this->real = real;
this->imaginary = imaginary;
this->modInteger = real * real + imaginary * imaginary;
}
};
bool compareComplexNumbers(const ComplexNumber& a, const ComplexNumber& b) {
if (a.modInteger != b.modInteger) {
return a.modInteger < b.modInteger;
} else if (a.real != b.real) {
return a.real < b.real;
} else {
return a.imaginary < b.imaginary;
}
}
int main() {
std::vector<ComplexNumber> complexNumbers;
int id, real, imaginary;
while (std::cin >> id && id != 0) {
std::cin >> real >> imaginary;
complexNumbers.push_back(ComplexNumber(id, real, imaginary));
}
std::sort(complexNumbers.begin(), complexNumbers.end(), compareComplexNumbers);
for (const auto& complexNumber : complexNumbers) {
std::cout << complexNumber.id << " ";
}
return 0;
}
```
以上代码首先定义了一个结构体 `ComplexNumber`,用于表示复数,包含编号、实部、虚部和模的整数部分。然后定义了一个比较函数 `compareComplexNumbers`,用于按要求对复数进行排序。接下来,在主函数中,通过循环读取输入的复数信息,并将其存储在一个向量 `complexNumbers` 中。最后,使用 `std::sort` 函数对向量中的复数进行排序,并依次输出排序后的复数编号。
你可以使用以上代码来解决你的问题。希望能帮到你!
阅读全文
相关推荐
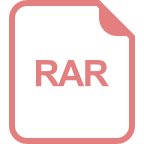
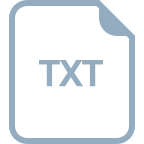















