给一个详细的示例
时间: 2024-02-17 15:04:41 浏览: 23
好的,下面是一个更详细的使用 libssh 库实现 SFTP 的示例,包括连接远程服务器、上传文件、下载文件、删除文件和创建目录等操作。
```c++
#include <iostream>
#include <string>
#include <vector>
#include <libssh/libssh.h>
#include <libssh/sftp.h>
// SFTP 文件信息结构体
struct SftpFileInfo {
std::string name; // 文件名
bool is_directory; // 是否为目录
uint64_t size; // 文件大小
};
// 获取 SFTP 服务器上指定路径下的所有文件和目录(不包括 . 和 ..)
std::vector<SftpFileInfo> GetSftpFileList(sftp_session sftp, const std::string& path) {
std::vector<SftpFileInfo> file_list;
sftp_dir dir = sftp_opendir(sftp, path.c_str());
if (dir == nullptr) {
return file_list;
}
sftp_attributes attributes;
while ((attributes = sftp_readdir(sftp, dir)) != nullptr) {
if (std::string(attributes->name) == "." || std::string(attributes->name) == "..") {
continue;
}
SftpFileInfo file_info;
file_info.name = attributes->name;
file_info.is_directory = (attributes->permissions & S_IFDIR) == S_IFDIR;
file_info.size = attributes->size;
file_list.push_back(file_info);
}
sftp_closedir(dir);
return file_list;
}
// 判断 SFTP 服务器上指定路径下的文件或目录是否存在
bool IsSftpFileExist(sftp_session sftp, const std::string& path) {
sftp_attributes attributes = sftp_stat(sftp, path.c_str());
return attributes != nullptr;
}
// 上传文件到 SFTP 服务器
bool UploadFileToSftp(sftp_session sftp, const std::string& local_path, const std::string& remote_path) {
FILE* local_file = fopen(local_path.c_str(), "rb");
if (local_file == nullptr) {
std::cerr << "open local file failed" << std::endl;
return false;
}
sftp_file remote_file = sftp_open(sftp, remote_path.c_str(), O_WRONLY | O_CREAT, S_IRWXU);
if (remote_file == nullptr) {
std::cerr << "open remote file failed" << std::endl;
fclose(local_file);
return false;
}
char buffer[1024];
int bytes_read = 0;
while ((bytes_read = fread(buffer, 1, sizeof(buffer), local_file)) > 0) {
sftp_write(remote_file, buffer, bytes_read);
}
fclose(local_file);
sftp_close(remote_file);
return true;
}
// 下载 SFTP 服务器上的文件到本地
bool DownloadFileFromSftp(sftp_session sftp, const std::string& remote_path, const std::string& local_path) {
sftp_file remote_file = sftp_open(sftp, remote_path.c_str(), O_RDONLY, 0);
if (remote_file == nullptr) {
std::cerr << "open remote file failed" << std::endl;
return false;
}
FILE* local_file = fopen(local_path.c_str(), "wb");
if (local_file == nullptr) {
std::cerr << "create local file failed" << std::endl;
sftp_close(remote_file);
return false;
}
char buffer[1024];
int bytes_read = 0;
while ((bytes_read = sftp_read(remote_file, buffer, sizeof(buffer))) > 0) {
fwrite(buffer, 1, bytes_read, local_file);
}
fclose(local_file);
sftp_close(remote_file);
return true;
}
// 删除 SFTP 服务器上的文件或目录
bool RemoveSftpFileOrDirectory(sftp_session sftp, const std::string& path) {
int result = sftp_unlink(sftp, path.c_str());
if (result == SSH_ERROR) {
std::cerr << "remove remote file or directory failed" << std::endl;
return false;
}
return true;
}
// 创建 SFTP 服务器上的目录
bool CreateSftpDirectory(sftp_session sftp, const std::string& path) {
int result = sftp_mkdir(sftp, path.c_str(), S_IRWXU);
if (result == SSH_ERROR) {
std::cerr << "create remote directory failed" << std::endl;
return false;
}
return true;
}
int main() {
// 初始化 libssh 库
ssh_init();
// 连接 SFTP 服务器
ssh_session session = ssh_new();
ssh_options_set(session, SSH_OPTIONS_HOST, "sftp.example.com");
ssh_options_set(session, SSH_OPTIONS_PORT, 22);
ssh_options_set(session, SSH_OPTIONS_USER, "username");
ssh_connect(session);
int auth_result = ssh_userauth_password(session, nullptr, "password");
if (auth_result != SSH_AUTH_SUCCESS) {
std::cerr << "sftp login failed" << std::endl;
return 1;
}
// 打开 SFTP 会话
sftp_session sftp = sftp_new(session);
sftp_init(sftp);
// 获取远程目录下的文件列表
std::vector<SftpFileInfo> file_list = GetSftpFileList(sftp, "/remote/path");
// 判断远程文件是否存在
bool is_exist = IsSftpFileExist(sftp, "/remote/path/file.txt");
// 上传本地文件到远程服务器
bool upload_result = UploadFileToSftp(sftp, "/local/path/file.txt", "/remote/path/file.txt");
// 从远程服务器下载文件到本地
bool download_result = DownloadFileFromSftp(sftp, "/remote/path/file.txt", "/local/path/file.txt");
// 删除远程文件或目录
bool remove_result = RemoveSftpFileOrDirectory(sftp, "/remote/path/file.txt");
// 在远程服务器上创建目录
bool create_result = CreateSftpDirectory(sftp, "/remote/path/new_directory");
// 关闭 SFTP 会话和 SSH 连接
sftp_free(sftp);
ssh_disconnect(session);
ssh_free(session);
return 0;
}
```
需要注意的是,上述示例中的各个操作都只是简单实现,实际使用中还需要进行相应的异常处理和错误码处理。同时,由于 SFTP 协议的不同实现可能存在差异,因此在实际应用中还需要根据具体情况进行调整。
相关推荐
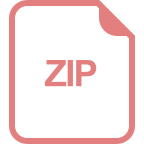
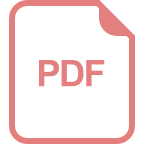















