用c语言写这个基于stm32的4路电阻温度测量程序
时间: 2024-03-08 13:46:09 浏览: 13
好的,我可以为您提供一份基于STM32的4路电阻温度测量程序的C语言代码示例。请注意,由于我无法直接访问STM32硬件,因此这只是一个基本的示例代码,您可能需要根据您的具体硬件配置和需求进行修改。
```
#include "stm32f4xx.h"
#include "stdio.h"
#include "stdlib.h"
#define ADC1_DR_ADDRESS ((uint32_t)0x4001204C)
float temp[4];
void ADC1_Init(void);
void GPIO_Init(void);
void USART1_Init(void);
void USART1_puts(char* s);
int main(void)
{
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_DMA2, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
GPIO_Init();
ADC1_Init();
USART1_Init();
while (1)
{
ADC_SoftwareStartConv(ADC1);
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
temp[0] = ((ADC_GetConversionValue(ADC1) * 3.3 / 4096 - 0.76) / 0.0025) + 25;
ADC_SoftwareStartConv(ADC1);
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
temp[1] = ((ADC_GetConversionValue(ADC1) * 3.3 / 4096 - 0.76) / 0.0025) + 25;
ADC_SoftwareStartConv(ADC1);
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
temp[2] = ((ADC_GetConversionValue(ADC1) * 3.3 / 4096 - 0.76) / 0.0025) + 25;
ADC_SoftwareStartConv(ADC1);
while(!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
temp[3] = ((ADC_GetConversionValue(ADC1) * 3.3 / 4096 - 0.76) / 0.0025) + 25;
char buffer[100];
sprintf(buffer, "Temp1: %.2f C\r\nTemp2: %.2f C\r\nTemp3: %.2f C\r\nTemp4: %.2f C\r\n", temp[0], temp[1], temp[2], temp[3]);
USART1_puts(buffer);
delay_ms(1000);
}
}
void ADC1_Init(void)
{
ADC_CommonInitTypeDef ADC_CommonInitStructure;
ADC_InitTypeDef ADC_InitStructure;
ADC_CommonInitStructure.ADC_Mode = ADC_Mode_Independent;
ADC_CommonInitStructure.ADC_Prescaler = ADC_Prescaler_Div2;
ADC_CommonInitStructure.ADC_DMAAccessMode = ADC_DMAAccessMode_Disabled;
ADC_CommonInitStructure.ADC_TwoSamplingDelay = ADC_TwoSamplingDelay_5Cycles;
ADC_CommonInit(&ADC_CommonInitStructure);
ADC_InitStructure.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStructure.ADC_ScanConvMode = ENABLE;
ADC_InitStructure.ADC_ContinuousConvMode = DISABLE;
ADC_InitStructure.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfConversion = 4;
ADC_Init(ADC1, &ADC_InitStructure);
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_480Cycles);
ADC_RegularChannelConfig(ADC1, ADC_Channel_1, 2, ADC_SampleTime_480Cycles);
ADC_RegularChannelConfig(ADC1, ADC_Channel_2, 3, ADC_SampleTime_480Cycles);
ADC_RegularChannelConfig(ADC1, ADC_Channel_3, 4, ADC_SampleTime_480Cycles);
ADC_DMARequestAfterLastTransferCmd(ADC1, ENABLE);
ADC_DMACmd(ADC1, ENABLE);
ADC_Cmd(ADC1, ENABLE);
}
void GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource9, GPIO_AF_USART1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource10, GPIO_AF_USART1);
}
void USART1_Init(void)
{
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART1_puts(char* s)
{
while(*s)
{
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, *s++);
}
}
void delay_ms(uint32_t ms)
{
volatile uint32_t nCount;
RCC_ClocksTypeDef RCC_Clocks;
RCC_GetClocksFreq(&RCC_Clocks);
nCount=(RCC_Clocks.HCLK_Frequency/10000)*ms;
for(; nCount!=0; nCount--);
}
```
上述代码使用了4个通道进行ADC转换,每个通道的输入电压都通过电阻分压器接在了ADC的输入端口。程序中的ADC采样时间、参考电压和电压分压器的参数都需要根据具体的硬件配置进行修改。在转换完成后,程序通过USART1把测量数据发送到串口终端。
以上代码仅供参考,具体实现方式可能因硬件和需求的不同而有所不同,您需要根据具体情况进行修改和调试。
相关推荐
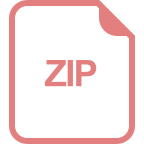
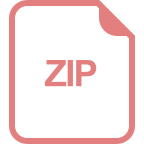
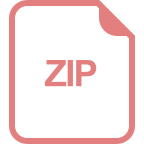
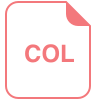
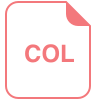
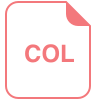
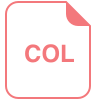
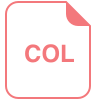









