opencv 使用calib3D确认相机位姿代码c++
时间: 2023-09-18 20:05:52 浏览: 105
### 回答1:
以下是使用OpenCV中的calib3d库函数来确定相机位姿的示例代码:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main()
{
// 读取相机内参数和畸变系数
Mat cameraMatrix = Mat::eye(3, 3, CV_64F);
Mat distCoeffs = Mat::zeros(5, 1, CV_64F);
FileStorage fs("calibration.xml", FileStorage::READ);
if (!fs.isOpened())
{
cerr << "Failed to open calibration.xml" << endl;
return -1;
}
fs["camera_matrix"] >> cameraMatrix;
fs["distortion_coefficients"] >> distCoeffs;
fs.release();
// 读取图像和物体点
Mat img = imread("image.jpg");
vector<Point3f> objectPoints;
objectPoints.push_back(Point3f(0, 0, 0));
objectPoints.push_back(Point3f(1, 0, 0));
objectPoints.push_back(Point3f(0, 1, 0));
objectPoints.push_back(Point3f(0, 0, 1));
// 检测图像中的特征点
vector<Point2f> imagePoints;
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
goodFeaturesToTrack(gray, imagePoints, 4, 0.01, 10);
// 计算相机位姿
Mat rvec, tvec;
solvePnP(objectPoints, imagePoints, cameraMatrix, distCoeffs, rvec, tvec);
// 输出相机位姿
Mat R;
Rodrigues(rvec, R);
cout << "Rotation Matrix:" << endl << R << endl;
cout << "Translation Vector:" << endl << tvec << endl;
return 0;
}
```
这段代码假定已经对相机进行了标定,并且内参数和畸变系数存储在名为“calibration.xml”的文件中。然后,代码读取一张图像和一组物体点,并使用`goodFeaturesToTrack`函数检测图像中的特征点。最后,`solvePnP`函数使用物体点和相应的图像点来计算相机的位姿(旋转和平移向量)。`Rodrigues`函数将旋转向量转换为旋转矩阵。最终,程序将输出旋转矩阵和平移向量。
### 回答2:
在使用Calib3D库来确认相机姿态时,需要使用OpenCV的C++代码。以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
int main() {
// 读取相机内参数矩阵和畸变参数
cv::Mat cameraMatrix, distCoeffs;
cv::FileStorage fs("camera_calib.xml", cv::FileStorage::READ);
fs["camera_matrix"] >> cameraMatrix;
fs["dist_coeffs"] >> distCoeffs;
fs.release();
// 读取图像和标定板参数
cv::Mat image = cv::imread("calibration_image.jpg");
cv::Size boardSize(9, 6);
std::vector<cv::Point2f> corners;
bool patternFound = cv::findChessboardCorners(image, boardSize, corners);
// 通过棋盘角点计算相机位姿
cv::Mat rvec, tvec;
if (patternFound) {
cv::solvePnP(cv::Mat(boardSize.width * boardSize.height, 3, CV_32FC1, corners.data()),
cv::Mat(3, 1, CV_32FC1, cv::Scalar::all(0)),
cameraMatrix, distCoeffs, rvec, tvec);
std::cout << "Rotation Vector: " << rvec << std::endl;
std::cout << "Translation Vector: " << tvec << std::endl;
} else {
std::cout << "Pattern not found!" << std::endl;
}
return 0;
}
```
上述代码首先加载相机的内参数矩阵和畸变参数,然后读取用于标定的图像,以及标定板的参数。接下来使用`findChessboardCorners`函数检测图像中的棋盘角点。
如果检测成功,就使用`solvePnP`函数来计算相机的旋转向量(rvec)和平移向量(tvec),这些向量可以用来描述相机在世界坐标系中的位置。
最后,通过这两个向量,我们可以得到相机的位姿。在上述代码中,最终的位姿结果会打印在控制台上。如果图像中的棋盘角点没有被成功检测到,则会输出"Pattern not found!"。
需要注意的是,上述代码仅仅是一个简单的示例,具体的操作和参数设置可能需要根据实际情况进行调整。
### 回答3:
在使用OpenCV中的calib3D库确认相机位姿时,可以使用以下的C++代码:
```cpp
#include <opencv2/opencv.hpp>
int main()
{
// 加载相机参数和参考图像和当前图像
cv::Mat cameraMatrix, distCoeffs, imgRef, imgCur;
cv::FileStorage fs("camera_params.xml", cv::FileStorage::READ);
fs["camera_matrix"] >> cameraMatrix;
fs["distortion_coefficients"] >> distCoeffs;
fs.release();
imgRef = cv::imread("reference_image.jpg", cv::IMREAD_GRAYSCALE);
imgCur = cv::imread("current_image.jpg", cv::IMREAD_GRAYSCALE);
// 特征点匹配
std::vector<cv::KeyPoint> keypointsRef, keypointsCur;
cv::Ptr<cv::FeatureDetector> detector = cv::ORB::create();
detector->detect(imgRef, keypointsRef);
detector->detect(imgCur, keypointsCur);
cv::Ptr<cv::DescriptorExtractor> extractor = cv::ORB::create();
cv::Mat descriptorsRef, descriptorsCur;
extractor->compute(imgRef, keypointsRef, descriptorsRef);
extractor->compute(imgCur, keypointsCur, descriptorsCur);
// 特征点匹配算法
cv::BFMatcher matcher(cv::NORM_HAMMING);
std::vector<cv::DMatch> matches;
matcher.match(descriptorsRef, descriptorsCur, matches);
// 筛选出最好的匹配
std::sort(matches.begin(), matches.end());
const int numGoodMatches = matches.size() * 0.15;
matches.erase(matches.begin() + numGoodMatches, matches.end());
// 提取匹配的特征点
std::vector<cv::Point2f> obj, scene;
for (size_t i = 0; i < matches.size(); i++)
{
obj.push_back(keypointsRef[matches[i].queryIdx].pt);
scene.push_back(keypointsCur[matches[i].trainIdx].pt);
}
// 计算相机的旋转和平移矩阵
cv::Mat rvec, tvec;
cv::solvePnP(obj, scene, cameraMatrix, distCoeffs, rvec, tvec);
// 输出相机位姿
cv::Mat rotMat;
cv::Rodrigues(rvec, rotMat);
std::cout << "旋转矩阵:" << std::endl << rotMat << std::endl;
std::cout << "平移向量:" << std::endl << tvec << std::endl;
return 0;
}
```
代码中,首先加载相机的内参矩阵和畸变系数,以及参考图像和当前图像。然后使用ORB特征点检测和描述子提取算法提取两张图像的特征点和描述子,并使用特征点匹配算法找到最佳匹配的特征点。接下来,利用求解PnP问题的算法计算出相机的旋转和平移矩阵。最后,通过Rodrigues变换将旋转向量转换为旋转矩阵,并输出相机位姿的旋转矩阵和平移向量。
这段代码就是使用OpenCV中calib3D库确认相机位姿的一个简单示例。
相关推荐
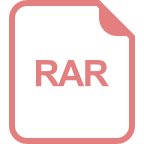















