如何在gru中添加自注意力机制
时间: 2023-12-03 14:58:20 浏览: 34
在GRU中添加自注意力机制需要进行以下步骤:
1. 定义注意力权重函数
注意力权重函数可以使用多种方式定义,其中一种是通过计算输入序列中每个时间步与上一个隐藏状态之间的相似度,然后将相似度通过softmax函数进行归一化得到注意力权重。具体可参考以下代码实现:
```python
def attention(query, key, value):
# query: (batch_size, hidden_size)
# key: (seq_len, batch_size, hidden_size)
# value: (seq_len, batch_size, hidden_size)
energy = torch.matmul(key, query.unsqueeze(2)).squeeze(2) # (seq_len, batch_size)
attention_weights = F.softmax(energy, dim=0) # (seq_len, batch_size)
context_vector = torch.matmul(attention_weights.unsqueeze(1), value.transpose(0, 1)).squeeze(1) # (batch_size, hidden_size)
return context_vector
```
2. 在GRU中调用注意力权重函数
在GRU的每个时间步中,计算出当前隐藏状态和输入序列中每个时间步的注意力权重,然后将注意力权重加权平均得到上下文向量,将上下文向量与当前隐藏状态进行拼接,作为当前时间步的输入。具体可参考以下代码实现:
```python
class AttentionGRU(nn.Module):
def __init__(self, input_size, hidden_size):
super(AttentionGRU, self).__init__()
self.input_size = input_size
self.hidden_size = hidden_size
self.gru = nn.GRU(input_size, hidden_size, batch_first=True)
self.attn = nn.Linear(hidden_size, hidden_size)
def forward(self, input_seq, hidden_state):
seq_len = input_seq.size(1)
key = self.attn(hidden_state[-1]).unsqueeze(0).repeat(seq_len, 1, 1) # (seq_len, batch_size, hidden_size)
value = input_seq.transpose(0, 1) # (seq_len, batch_size, input_size)
context_vector = attention(hidden_state[-1], key, value) # (batch_size, hidden_size)
gru_input = torch.cat([input_seq, context_vector.unsqueeze(1).repeat(1, seq_len, 1)], dim=-1) # (batch_size, seq_len, input_size+hidden_size)
output, hidden_state = self.gru(gru_input, hidden_state)
return output, hidden_state
```
上述代码中,`AttentionGRU` 继承自 `nn.Module`,其中 `self.gru` 是一个标准的GRU单元,`self.attn` 是用于计算注意力权重的线性层。在前向传播时,先将输入序列 `input_seq` 和上一个隐藏状态 `hidden_state` 传入注意力权重函数 `attention` 中,得到上下文向量 `context_vector`。然后将输入序列和上下文向量拼接起来,作为GRU的输入。最后返回GRU的输出和新的隐藏状态。
这样就可以在GRU中添加自注意力机制了。
相关推荐
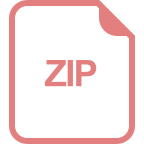
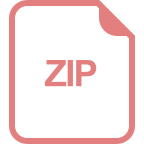
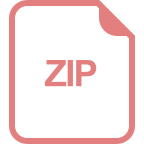














