thymeleaf+bootstrap5 发送ajax请求
时间: 2023-05-04 17:06:30 浏览: 595
Thymeleaf是一种Java模板引擎,而Bootstrap是一种前端框架,可以使Web开发更加高效。要使用Thymeleaf和Bootstrap5发送Ajax请求,我们可以使用jQuery的Ajax函数来实现。
首先,我们需要在HTML文件中引入jQuery库和Bootstrap样式库。然后,在需要发送Ajax请求的按钮或链接上添加一个ID或特定的类,并使用JavaScript代码将其与Ajax事件绑定。在Ajax事件中,我们可以指定请求类型、URL、数据等,还可以定义成功或失败时要执行的代码。
对于Thymeleaf,我们可以使用其内置的表达式语言将数据绑定到HTML元素中,例如将表格中的数据使用循环结构进行填充,或将表单的数据传递到控制器中。
具体而言,我们可以使用如下代码来实现Thymeleaf Bootstrap5发送Ajax请求:
HTML代码:
```html
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Thymeleaf Bootstrap5 Ajax Request Demo</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.1.1/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h2>Thymeleaf Bootstrap5 Ajax Request Demo</h2>
<table class="table table-striped table-bordered table-hover">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr th:each="user: ${users}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.age}"></td>
<td>
<a href="javascript:void(0)" class="btn btn-info btn-xs edit-btn" th:data-id="${user.id}">Edit</a>
<a href="javascript:void(0)" class="btn btn-danger btn-xs delete-btn" th:data-id="${user.id}">Delete</a>
</td>
</tr>
</tbody>
</table>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.1.1/js/bootstrap.bundle.min.js"></script>
<script>
$(document).ready(function () {
// Edit button click event
$('.edit-btn').click(function () {
var id = $(this).data('id');
$.ajax({
type: "GET",
url: "/user/" + id,
success: function (data) {
$('#id').val(data.id);
$('#name').val(data.name);
$('#age').val(data.age);
$('#editModal').modal('show');
},
error: function (jqXHR, textStatus, errorThrown) {
alert('Error getting user by ID');
}
});
});
// Delete button click event
$('.delete-btn').click(function () {
var id = $(this).data('id');
if (confirm('Are you sure you want to delete this user?')) {
$.ajax({
type: "DELETE",
url: "/user/" + id,
success: function (data) {
window.location.reload();
},
error: function (jqXHR, textStatus, errorThrown) {
alert('Error deleting user');
}
});
}
});
});
</script>
</body>
</html>
```
这里我们为Edit按钮和Delete按钮分别添加了edit-btn和delete-btn类,并为其设置了数据属性th:data-id,用于记录需要编辑或删除的用户ID。在JavaScript代码中,我们使用jQuery的Ajax函数分别为这两个按钮绑定了点击事件,并在事件中分别发送了GET和DELETE类型的Ajax请求,获取或删除对应的用户数据。
需要注意的是,我们使用了Thymeleaf的内置表达式语言将数据渲染到了HTML中,并在用户单击Edit按钮时使用了Bootstrap的Modal组件来显示编辑面板。此外,我们还为删除按钮添加了一个确认框,以防止误操作。
总的来说,使用Thymeleaf Bootstrap5发送Ajax请求非常方便,能够大大提高Web开发效率和用户体验。
阅读全文
相关推荐
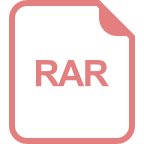
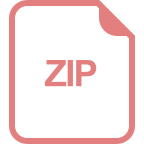
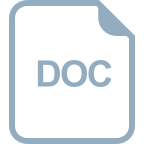
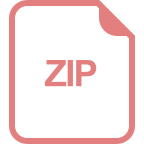
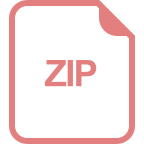
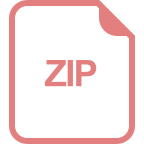
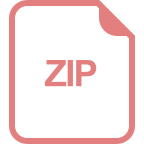
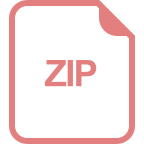
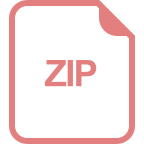

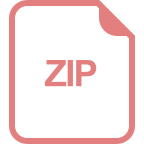
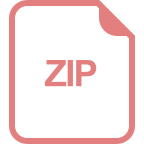
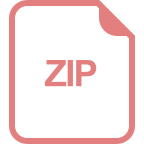
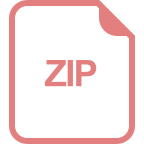
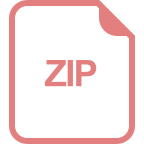


