用c语言或者c++语言
时间: 2024-03-10 19:44:25 浏览: 88
好的,下面是用C++语言实现一个简单的Thrift服务的代码示例:
首先,需要安装Thrift编译器,并且编写一个Thrift定义文件,例如`auth.thrift`:
```
namespace cpp com.example.auth
struct User {
1: required string username,
2: required string password
}
exception AuthException {
1: required string message
}
service AuthService {
string authenticate(1: User user) throws (1: AuthException ex)
}
```
然后,使用Thrift编译器生成C++代码:
```
thrift -r --gen cpp auth.thrift
```
这会生成一个`gen-cpp`目录,其中包含了自动生成的C++代码。现在,可以使用这些代码实现一个`AuthServiceHandler`类,用于处理客户端的请求,例如:
```c++
#include "gen-cpp/AuthService.h"
using namespace std;
using namespace com::example::auth;
class AuthServiceHandler : virtual public AuthServiceIf {
public:
string authenticate(const User& user) {
// TODO: 实现认证逻辑
return "token";
}
};
```
在这个示例中,`AuthServiceHandler`类实现了`AuthServiceIf`接口,并重载了`authenticate`方法,用于实现认证逻辑。在实际应用中,可以使用数据库或其他方式来验证用户身份。
最后,可以使用Thrift生成的服务器代码启动一个Thrift服务器,并将`AuthServiceHandler`类作为参数传递给服务器,例如:
```c++
#include <thrift/server/TSimpleServer.h>
#include <thrift/transport/TServerSocket.h>
#include <thrift/transport/TBufferTransports.h>
#include "gen-cpp/AuthService.h"
using namespace std;
using namespace com::example::auth;
using namespace apache::thrift;
using namespace apache::thrift::server;
using namespace apache::thrift::transport;
int main() {
int port = 9090;
shared_ptr<AuthServiceHandler> handler(new AuthServiceHandler());
shared_ptr<TProcessor> processor(new AuthServiceProcessor(handler));
shared_ptr<TServerTransport> serverTransport(new TServerSocket(port));
shared_ptr<TTransportFactory> transportFactory(new TBufferedTransportFactory());
shared_ptr<TProtocolFactory> protocolFactory(new TBinaryProtocolFactory());
TSimpleServer server(processor, serverTransport, transportFactory, protocolFactory);
server.serve();
return 0;
}
```
在这个示例中,使用Thrift生成的`AuthServiceProcessor`类将`AuthServiceHandler`类包装成一个Thrift服务处理器,并将其作为参数传递给`TSimpleServer`服务器。最后,调用`server.serve()`方法启动Thrift服务器。
当Thrift服务器运行后,就可以使用Thrift生成的客户端代码来调用`AuthService`服务的`authenticate`方法了。客户端代码可以使用C++、Java、Python等语言来编写,具体实现方式可以参考Thrift官方文档。
阅读全文
相关推荐
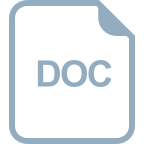
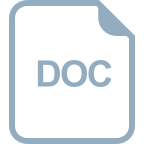
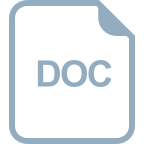
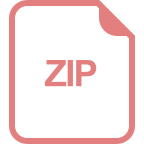
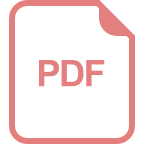
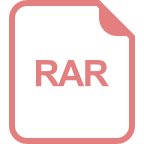
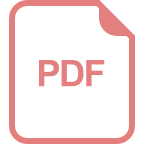
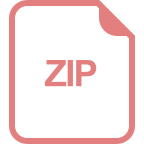
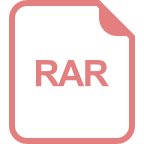
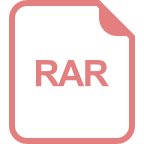
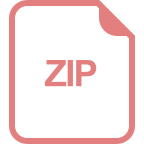
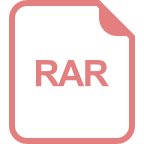
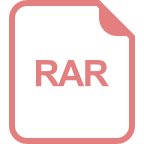
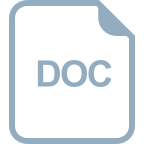
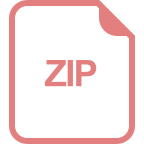
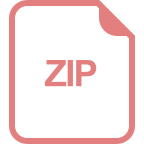
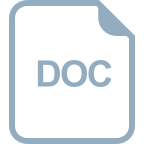
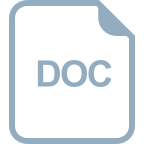
