银行家算法c语言设计
时间: 2024-05-22 09:08:37 浏览: 164
银行家算法是一种用于避免死锁的算法,主要用于多个进程共享有限数量的资源的场景中。在该算法中,系统维护了每种资源的可用数量和每个进程还需要的资源数量,通过分配资源给需要资源的进程以及释放已经占用资源的进程来避免死锁的发生。
银行家算法的C语言设计可以参考以下步骤:
1. 定义系统中资源的种类及每种资源的总数量。
2. 定义系统中每个进程需要的资源数量和已经占用的资源数量。
3. 编写函数来判断是否存在安全序列,即是否能够分配资源避免死锁。
4. 编写函数来分配资源给请求资源的进程。
5. 编写函数来释放已经占用资源的进程。
具体实现可以根据具体情况进行调整和完善。如果需要更具体的代码实现,请提供更详细的需求和背景信息,我可以为您提供更详细的帮助。
相关问题
银行家算法 C语言设计交互界面 codeblock
以下是一个简单的银行家算法C语言实现的交互界面,使用CodeBlocks编写:
```c
#include <stdio.h>
#define MAX_RESOURCES 10
int available[MAX_RESOURCES];
int allocation[MAX_RESOURCES][MAX_RESOURCES];
int need[MAX_RESOURCES][MAX_RESOURCES];
int processes_count;
void init() {
printf("Enter the number of processes: ");
scanf("%d", &processes_count);
printf("Enter the number of available resources: ");
int resources_count;
scanf("%d", &resources_count);
printf("Enter the available resources:\n");
for (int i = 0; i < resources_count; i++) {
scanf("%d", &available[i]);
}
printf("Enter the allocation matrix:\n");
for (int i = 0; i < processes_count; i++) {
for (int j = 0; j < resources_count; j++) {
scanf("%d", &allocation[i][j]);
need[i][j] = allocation[i][j];
available[j] -= allocation[i][j];
}
}
printf("Initialization complete.\n");
}
int request_resources(int process_id, int request[]) {
for (int i = 0; i < MAX_RESOURCES; i++) {
if (request[i] > need[process_id][i]) {
printf("Request exceeds need.\n");
return 0;
}
if (request[i] > available[i]) {
printf("Request exceeds available resources.\n");
return 0;
}
}
for (int i = 0; i < MAX_RESOURCES; i++) {
available[i] -= request[i];
allocation[process_id][i] += request[i];
need[process_id][i] -= request[i];
}
printf("Request granted.\n");
return 1;
}
int release_resources(int process_id, int release[]) {
for (int i = 0; i < MAX_RESOURCES; i++) {
if (release[i] > allocation[process_id][i]) {
printf("Release exceeds allocation.\n");
return 0;
}
}
for (int i = 0; i < MAX_RESOURCES; i++) {
available[i] += release[i];
allocation[process_id][i] -= release[i];
need[process_id][i] += release[i];
}
printf("Resources released.\n");
return 1;
}
void print_state() {
printf("Available resources:\n");
for (int i = 0; i < MAX_RESOURCES; i++) {
printf("%d ", available[i]);
}
printf("\n");
printf("Allocation matrix:\n");
for (int i = 0; i < processes_count; i++) {
for (int j = 0; j < MAX_RESOURCES; j++) {
printf("%d ", allocation[i][j]);
}
printf("\n");
}
printf("Need matrix:\n");
for (int i = 0; i < processes_count; i++) {
for (int j = 0; j < MAX_RESOURCES; j++) {
printf("%d ", need[i][j]);
}
printf("\n");
}
}
int main() {
init();
while (1) {
printf("\nEnter command (request, release, state, quit): ");
char command[10];
scanf("%s", command);
if (strcmp(command, "request") == 0) {
int process_id;
printf("Enter process id: ");
scanf("%d", &process_id);
int request[MAX_RESOURCES];
printf("Enter request: ");
for (int i = 0; i < MAX_RESOURCES; i++) {
scanf("%d", &request[i]);
}
request_resources(process_id, request);
} else if (strcmp(command, "release") == 0) {
int process_id;
printf("Enter process id: ");
scanf("%d", &process_id);
int release[MAX_RESOURCES];
printf("Enter release: ");
for (int i = 0; i < MAX_RESOURCES; i++) {
scanf("%d", &release[i]);
}
release_resources(process_id, release);
} else if (strcmp(command, "state") == 0) {
print_state();
} else if (strcmp(command, "quit") == 0) {
break;
} else {
printf("Invalid command.\n");
}
}
return 0;
}
```
该程序实现了银行家算法的初始化、请求资源、释放资源和打印当前状态等功能。用户可以通过命令行输入不同的命令与程序进行交互。
银行家算法 C语言设计交互界面 codeblocks20.23
以下是银行家算法在 C 语言中的代码,包含了交互界面:
```c
#include <stdio.h>
int main() {
int n, m, i, j, k, p;
int available[10], max[10][10], allocation[10][10], need[10][10];
int request[10], finish[10], work[10];
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the number of resources: ");
scanf("%d", &m);
printf("Enter the number of available resources: ");
for (i = 0; i < m; i++) {
scanf("%d", &available[i]);
}
printf("Enter the maximum demand of each process: ");
for (i = 0; i < n; i++) {
printf("For process %d: ", i + 1);
for (j = 0; j < m; j++) {
scanf("%d", &max[i][j]);
}
}
printf("Enter the allocation for each process: ");
for (i = 0; i < n; i++) {
printf("For process %d: ", i + 1);
for (j = 0; j < m; j++) {
scanf("%d", &allocation[i][j]);
need[i][j] = max[i][j] - allocation[i][j];
}
}
for (i = 0; i < n; i++) {
finish[i] = 0;
}
for (i = 0; i < m; i++) {
work[i] = available[i];
}
p = 0;
while (p < n) {
for (i = 0; i < n; i++) {
if (finish[i] == 0) {
for (j = 0; j < m; j++) {
if (need[i][j] > work[j]) {
break;
}
}
if (j == m) {
for (k = 0; k < m; k++) {
work[k] += allocation[i][k];
}
printf("Process %d completed\n", i + 1);
finish[i] = 1;
p++;
}
}
}
if (p == n) {
printf("System is in safe state\n");
}
else {
printf("System is in unsafe state\n");
}
printf("Do you want to request resources? (Enter 1 for yes, 0 for no): ");
scanf("%d", &j);
if (j == 1) {
printf("Enter the process number: ");
scanf("%d", &i);
printf("Enter the request: ");
for (k = 0; k < m; k++) {
scanf("%d", &request[k]);
}
for (k = 0; k < m; k++) {
if (request[k] > need[i][k]) {
printf("Error: Request is greater than need\n");
break;
}
if (request[k] > available[k]) {
printf("Error: Request is greater than available\n");
break;
}
}
if (k == m) {
for (k = 0; k < m; k++) {
available[k] -= request[k];
allocation[i][k] += request[k];
need[i][k] -= request[k];
}
}
}
}
return 0;
}
```
这个程序会先要求用户输入进程数、资源数和可用资源数,然后分别输入每个进程的最大需求和当前分配情况。程序会计算出每个进程的需求量,然后执行银行家算法,判断系统是否处于安全状态。如果不是,则会给用户一个选择是否请求资源的机会。如果用户选择请求资源,则需要输入进程号和请求量,然后程序会再次计算系统的状态并给出相应的输出。这个程序使用了 Code::Blocks 20.23 编译器,并在控制台中输出结果。
阅读全文
相关推荐




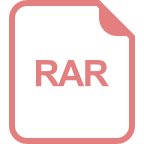


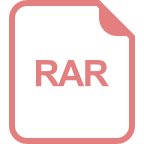
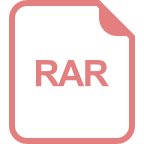




