银行家算法c语言代码设计思路
时间: 2023-07-30 17:12:55 浏览: 99
银行家算法是一种资源分配算法,其核心思想是在资源分配之前判断预分配后系统是否处于安全状态。下面是一个简单的C语言代码设计思路:
1. 定义进程结构体和资源结构体
首先,我们需要定义进程结构体和资源结构体,用来存储每个进程的资源需求、已分配和还需要的资源数量,以及系统中各个资源的总量和已分配数量。
```c
typedef struct {
int id; // 进程ID
int *max_resources; // 资源最大需求量
int *allocated_resources; // 已分配资源量
int *needed_resources; // 还需要资源量
} Process;
typedef struct {
int *available_resources; // 可用资源量
int *max_resources; // 资源总量
int *allocated_resources; // 已分配资源量
} Resource;
```
2. 实现安全状态检测函数
接下来,我们需要实现一个函数来检测系统是否处于安全状态。该函数接收系统中所有进程的信息和资源的信息作为参数,判断是否存在一个安全序列,如果存在,则返回1,否则返回0。
```c
int is_safe_state(Process *processes, Resource *resources, int num_processes, int num_resources) {
int *work = malloc(sizeof(int) * num_resources);
int *finish = malloc(sizeof(int) * num_processes);
int i, j, k;
// 初始化 work 数组和 finish 数组
for (i = 0; i < num_resources; i++) {
work[i] = resources->available_resources[i];
}
for (i = 0; i < num_processes; i++) {
finish[i] = 0;
}
// 模拟系统运行,直到所有进程都执行完
for (i = 0; i < num_processes; i++) {
for (j = 0; j < num_processes; j++) {
if (!finish[j]) {
// 判断进程 j 是否可以执行
int can_execute = 1;
for (k = 0; k < num_resources; k++) {
if (processes[j].needed_resources[k] > work[k]) {
can_execute = 0;
break;
}
}
if (can_execute) {
// 执行进程 j 并释放它占用的资源
finish[j] = 1;
for (k = 0; k < num_resources; k++) {
work[k] += processes[j].allocated_resources[k];
}
break;
}
}
}
if (j == num_processes) {
// 不存在可以执行的进程,系统不处于安全状态
free(work);
free(finish);
return 0;
}
}
// 所有进程都执行完了,系统处于安全状态
free(work);
free(finish);
return 1;
}
```
3. 实现资源分配函数
当系统接收到一个进程的资源申请请求时,需要判断是否可以满足该请求。如果可以满足,则修改进程的资源分配情况,并重新判断系统是否处于安全状态。如果不能满足,则需要等待其他进程释放资源或回收已分配资源等,来避免死锁的发生。
```c
int allocate_resources(Process *process, Resource *resources, int num_resources) {
int i;
// 判断进程的资源需求是否小于等于还需要的资源量
for (i = 0; i < num_resources; i++) {
if (process->max_resources[i] - process->allocated_resources[i] > resources->allocated_resources[i]) {
return 0;
}
}
// 分配资源,并重新判断系统是否处于安全状态
for (i = 0; i < num_resources; i++) {
resources->allocated_resources[i] -= process->max_resources[i];
process->allocated_resources[i] += process->max_resources[i];
process->needed_resources[i] -= process->max_resources[i];
}
if (is_safe_state(processes, resources, num_processes, num_resources)) {
return 1;
} else {
// 如果分配后系统不处于安全状态,则回收已分配资源
for (i = 0; i < num_resources; i++) {
resources->allocated_resources[i] += process->max_resources[i];
process->allocated_resources[i] -= process->max_resources[i];
process->needed_resources[i] += process->max_resources[i];
}
return 0;
}
}
```
4. 主函数
最后,我们需要编写一个主函数来模拟银行家算法的运行过程。在主函数中,我们需要初始化进程、资源和资源分配情况,接收用户的资源申请请求,并调用资源分配函数来判断是否可以满足该请求。
```c
int main() {
int num_processes, num_resources, i, j;
printf("请输入进程数和资源数:");
scanf("%d %d", &num_processes, &num_resources);
Process *processes = malloc(sizeof(Process) * num_processes);
Resource resources;
resources.available_resources = malloc(sizeof(int) * num_resources);
resources.max_resources = malloc(sizeof(int) * num_resources);
resources.allocated_resources = malloc(sizeof(int) * num_resources);
// 初始化进程、资源和资源分配情况
for (i = 0; i < num_processes; i++) {
processes[i].id = i;
processes[i].max_resources = malloc(sizeof(int) * num_resources);
processes[i].allocated_resources = malloc(sizeof(int) * num_resources);
processes[i].needed_resources = malloc(sizeof(int) * num_resources);
printf("请输入进程 %d 的资源最大需求量:", i);
for (j = 0; j < num_resources; j++) {
scanf("%d", &processes[i].max_resources[j]);
processes[i].needed_resources[j] = processes[i].max_resources[j];
}
}
printf("请输入资源总量:");
for (i = 0; i < num_resources; i++) {
scanf("%d", &resources.max_resources[i]);
resources.available_resources[i] = resources.max_resources[i];
resources.allocated_resources[i] = 0;
}
// 接收用户的资源申请请求
printf("请输入进程ID和资源申请量:");
int id, *request_resources;
request_resources = malloc(sizeof(int) * num_resources);
scanf("%d", &id);
for (i = 0; i < num_resources; i++) {
scanf("%d", &request_resources[i]);
processes[id].max_resources[i] = request_resources[i];
processes[id].needed_resources[i] -= request_resources[i];
}
// 判断是否可以分配资源
if (allocate_resources(&processes[id], &resources, num_resources)) {
printf("系统处于安全状态,已分配资源。\n");
} else {
printf("系统不处于安全状态,不能满足请求。\n");
}
// 释放内存
for (i = 0; i < num_processes; i++) {
free(processes[i].max_resources);
free(processes[i].allocated_resources);
free(processes[i].needed_resources);
}
free(processes);
free(resources.available_resources);
free(resources.max_resources);
free(resources.allocated_resources);
free(request_resources);
return 0;
}
```
以上是一个简单的银行家算法的C语言代码设计思路,仅供参考。
阅读全文
相关推荐
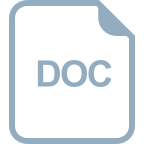
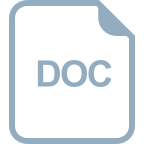
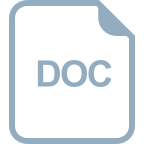

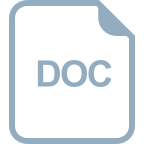
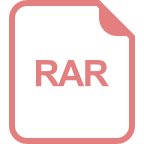
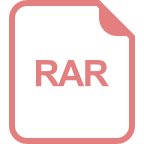
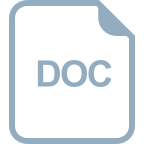
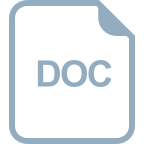
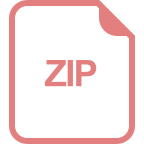
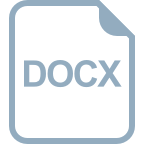
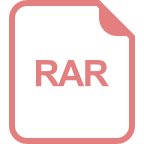
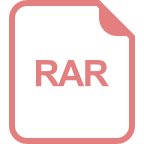
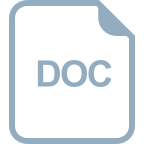
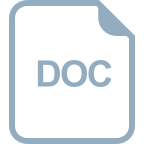
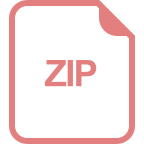
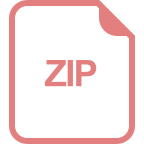