C++ 实现配置文件的读取写入
时间: 2023-09-26 07:07:53 浏览: 120
C++ 读取和写入配置文件可以使用标准库中的 `<fstream>` 头文件中提供的 `ifstream` 和 `ofstream` 类。
假设我们的配置文件格式为:
```
key1=value1
key2=value2
key3=value3
```
以下是读取配置文件的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <map>
int main() {
std::ifstream configFile("config.txt");
if (!configFile.is_open()) {
std::cerr << "Failed to open config file!" << std::endl;
return 1;
}
std::map<std::string, std::string> config;
std::string line;
while (std::getline(configFile, line)) {
size_t equalPos = line.find('=');
if (equalPos == std::string::npos) {
continue;
}
std::string key = line.substr(0, equalPos);
std::string value = line.substr(equalPos + 1);
config[key] = value;
}
configFile.close();
for (auto it = config.begin(); it != config.end(); ++it) {
std::cout << it->first << " = " << it->second << std::endl;
}
return 0;
}
```
以上代码首先打开 `config.txt` 文件,然后逐行读取文件内容,并将每一行按照等号分割为键和值。最后将所有键值对存储到一个 `std::map` 容器中,并输出所有配置项。
以下是写入配置文件的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <map>
int main() {
std::map<std::string, std::string> config = {
{"key1", "value1"},
{"key2", "value2"},
{"key3", "value3"}
};
std::ofstream configFile("config.txt");
if (!configFile.is_open()) {
std::cerr << "Failed to open config file!" << std::endl;
return 1;
}
for (auto it = config.begin(); it != config.end(); ++it) {
configFile << it->first << "=" << it->second << std::endl;
}
configFile.close();
return 0;
}
```
以上代码首先定义一个 `std::map` 容器存储所有配置项,然后打开 `config.txt` 文件并逐一写入每个配置项。最后关闭文件流。
需要注意的是,以上示例代码都没有进行错误处理,实际使用时应该考虑各种异常情况的处理。
相关推荐
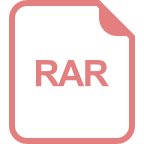














