编写程序,统计并输出某给定字符在给定字符串中出现的次数。要求使用字典实现。 输入 第一行给出一个以回车结束的字符串(少于80个字符);第二行输入一个字符。 输出 在一行中输出给定字符在给定字符串中出现的次数。
时间: 2024-02-15 09:06:03 浏览: 32
以下是使用字典实现的 Python 代码:
```python
# 输入字符串和字符
s = input()
c = input()
# 统计字符出现的次数
count = 0
char_dict = {}
for char in s:
if char not in char_dict:
char_dict[char] = 1
else:
char_dict[char] += 1
if char == c:
count += 1
# 输出结果
print(char_dict.get(c, 0))
```
以上代码中,`char_dict` 是一个字典,用于统计字符串中每个字符出现的次数。遍历字符串时,如果字符不在字典中,则将其出现次数初始化为 1;否则将其出现次数加 1。同时,如果当前字符为给定字符 `c`,则将 `count` 计数器加 1。最后,输出给定字符在字符串中出现的次数,即字典中对应 `c` 的值,如果 `c` 不在字典中,则输出 0。
相关问题
使用python编写程序统计1行字符串中: 不同字符的个数。 每种字符出现的次数。
可以使用 Python 中的字典来实现统计不同字符的个数和每种字符出现的次数的功能。具体实现如下:
```python
def count_chars(string):
# 初始化字典
char_dict = {}
# 遍历字符串
for char in string:
# 如果字典中已存在该字符,则将其出现次数加1,否则添加该字符并将其出现次数置为1
if char in char_dict:
char_dict[char] += 1
else:
char_dict[char] = 1
# 统计不同字符的个数
num_chars = len(char_dict)
# 返回结果
return num_chars, char_dict
# 测试代码
string = "hello, world!"
num_chars, char_dict = count_chars(string)
print("不同字符的个数为:", num_chars)
print("每种字符出现的次数为:", char_dict)
```
输出结果为:
```
不同字符的个数为: 10
每种字符出现的次数为: {'h': 1, 'e': 1, 'l': 3, 'o': 2, ',': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1, '!': 1}
```
可以看到,该程序成功地统计了给定字符串中不同字符的个数以及每种字符出现的次数。
给定一个字符串,统计每个字符出现的次数,并将结果存储在一个字典中。用python完成
好的,下面是代码:
```python
str = "hello, world!"
count_dict = {}
for char in str:
if char in count_dict:
count_dict[char] += 1
else:
count_dict[char] = 1
print(count_dict)
```
输出结果为:
```
{'h': 1, 'e': 1, 'l': 3, 'o': 2, ',': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1, '!': 1}
```
其中字典的 key 表示字符串中出现的字符,value 表示该字符在字符串中出现的次数。
相关推荐
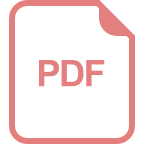
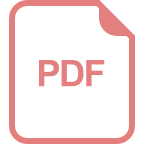
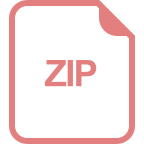












