C#List数组冒泡排序
时间: 2023-07-21 09:34:19 浏览: 102
以下是 C# 中使用冒泡排序算法对 List 数组进行排序的示例代码:
```csharp
int[] numbers = new int[] { 4, 2, 8, 3, 1, 9, 5, 7, 6 };
for (int i = 0; i < numbers.Length - 1; i++)
{
for (int j = 0; j < numbers.Length - i - 1; j++)
{
if (numbers[j] > numbers[j + 1])
{
int temp = numbers[j];
numbers[j] = numbers[j + 1];
numbers[j + 1] = temp;
}
}
}
foreach (int number in numbers)
{
Console.Write(number + " ");
}
```
在这个示例中,我们创建了一个包含整数的数组,然后使用嵌套的 for 循环遍历数组中的元素,比较相邻的两个元素的大小关系,如果前一个元素大于后一个元素,则交换它们的位置。这样,每一轮循环都会将数组中最大的元素移动到末尾。最后,我们使用 foreach 循环输出已排序的数组。
相关问题
C# list数组数据冒泡排序
在C#中,对List数组进行冒泡排序,需要遍历列表多次,比较相邻元素并交换它们的位置,直到整个列表有序。以下是冒泡排序的基本步骤:
```csharp
using System;
using System.Collections.Generic;
public class BubbleSortExample
{
public static void Main()
{
List<int> numbers = new List<int>() { 5, 3, 8, 6, 1 };
Console.WriteLine("Before sorting:");
PrintList(numbers);
// 冒泡排序
BubbleSort(numbers);
Console.WriteLine("\nAfter sorting:");
PrintList(numbers);
}
// 冒泡排序算法
public static void BubbleSort(List<int> list)
{
int n = list.Count;
for (int i = 0; i < n - 1; i++)
{
for (int j = 0; j < n - i - 1; j++)
{
if (list[j] > list[j + 1])
{
// 交换两个元素
int temp = list[j];
list[j] = list[j + 1];
list[j + 1] = temp;
}
}
}
}
// 打印列表
private static void PrintList(List<int> list)
{
foreach (int num in list)
{
Console.Write(num + " ");
}
Console.WriteLine();
}
}
```
在这个例子中,我们首先创建了一个包含无序整数的List,然后调用`BubbleSort`方法对其进行排序,并最后打印排序后的结果。
使用C#编写一段冒泡排序
使用C#编写一段冒泡排序的代码如下:
```csharp
public static void BubbleSort(int[] arr)
{
int n = arr.Length;
for (int i = 0; i < n - 1; i++)
{
for (int j = 0; j < n - i - 1; j++)
{
if (arr[j > arr[j + 1])
{
int temp = arr[j];
arr[j = arr[j + 1];
arr[j + 1 = temp;
}
}
}
}
```
在这段冒泡排序的代码中,首先定义了一个名为BubbleSort的静态方法,接受一个整数数组作为参数。然后,使用两个嵌套的for循环来进行排序。外层循环控制比较的轮数,内层循环用于相邻元素的比较和交换。如果当前元素大于下一个元素,则进行交换。最终,通过多次比较和交换,将数组中的元素按照从小到大的顺序排列。
注意,这只是冒泡排序的一种实现方式,还有其他的实现方式。此处提供的代码仅供参考。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [【排序算法】冒泡排序、简单选择排序、直接插入排序比较和分析](https://blog.csdn.net/baidu_33146219/article/details/126233101)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
阅读全文
相关推荐
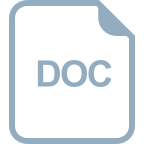
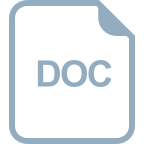
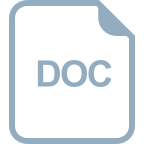
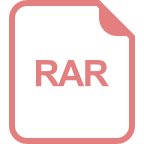
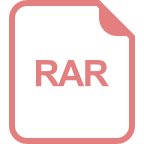
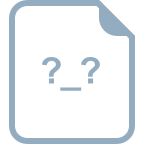
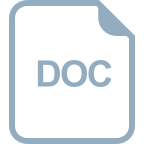
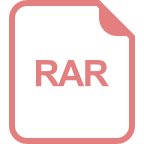
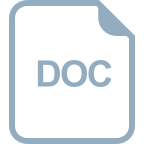
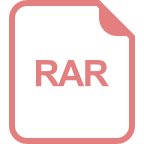
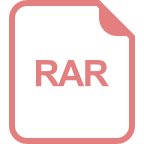
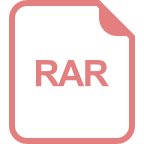
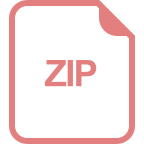
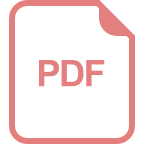
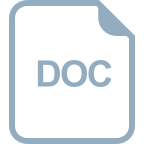
