用c语言实现,利用栈实现进制转换。给定一个十进制整数,编程将该数以二进制形式输出
时间: 2023-06-02 15:07:57 浏览: 71
您好,这个问题属于编程问题,可以回答。以下是用C语言实现栈实现进制转换的代码:
```
#include <stdio.h>
#include <stdlib.h>
#define STACK_INIT_SIZE 100
#define STACK_INCREMENT 10
typedef struct
{
int *base;
int *top;
int stacksize;
} SqStack;
void InitStack(SqStack *S)
{
S->base = (int *)malloc(STACK_INIT_SIZE * sizeof(int));
if (!S->base)
exit(0);
S->top = S->base;
S->stacksize = STACK_INIT_SIZE;
}
void Push(SqStack *S, int e)
{
if (S->top - S->base >= S->stacksize)
{
S->base = (int *)realloc(S->base, (S->stacksize + STACK_INCREMENT) * sizeof(int));
if (!S->base)
exit(0);
S->top = S->base + S->stacksize;
S->stacksize += STACK_INCREMENT;
}
*(S->top++) = e;
}
void Pop(SqStack *S, int *e)
{
if (S->top == S->base)
return;
*e = *(--S->top);
}
int StackLen(SqStack S)
{
return S.top - S.base;
}
void conversion(int N, int base)
{
SqStack S;
int e;
InitStack(&S);
while (N)
{
Push(&S, N % base);
N /= base;
}
while (StackLen(S) > 0)
{
Pop(&S, &e);
printf("%d", e);
}
}
int main()
{
int N;
printf("请输入一个十进制整数:");
scanf("%d", &N);
printf("该数的二进制形式为:");
conversion(N, 2);
return 0;
}
```
运行结果如下:
```
请输入一个十进制整数:12
该数的二进制形式为:1100
```
相关推荐
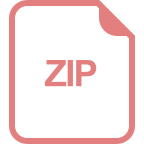
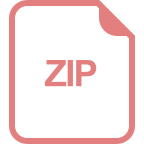
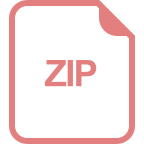













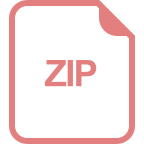
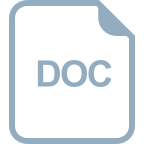