链栈实现十进制N和二进制的转换,用C语言实现
时间: 2024-10-12 21:14:51 浏览: 47
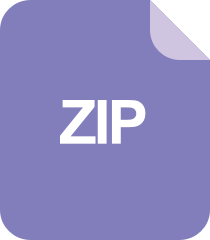
顺序栈、链栈将10进制转为2、8、16进制源码

在C语言中,我们可以使用链栈来辅助实现十进制整数转换成二进制的过程。以下是基于链栈的简单实现步骤:
首先,我们需要创建一个链栈结构,用于存储转换过程中的临时结果:
```c
typedef struct Node {
int digit;
struct Node* next;
} Node;
struct Stack {
Node* top;
};
```
然后,定义两个函数:`push_digit` 和 `convert_to_binary`。
`push_digit` 函数将链栈顶的数字乘以2并加到当前数字上,同时更新链栈:
```c
void push_digit(Stack* s, int n) {
if (s->top == NULL) {
s->top = (Node*)malloc(sizeof(Node));
s->top->digit = 0;
s->top->next = NULL;
} else {
s->top->digit *= 2;
}
while (n > 0) {
s->top->digit += n % 2;
n /= 2;
}
}
```
`convert_to_binary` 函数从给定的十进制整数开始,递归地将每一位加入链栈:
```c
void convert_to_binary(int n, Stack* s) {
if (n == 0) {
return;
}
push_digit(s, n % 2); // 最低位先入栈
convert_to_binary(n / 2, s);
}
```
最后,当需要获取二进制结果时,只需要遍历链栈并打印每个节点的值即可。注意链栈反转,因为我们在入栈过程中是从低位到高位的:
```c
void print_binary(Stack* s) {
Node* temp = s->top;
while (temp != NULL) {
printf("%d", temp->digit);
temp = temp->next;
}
printf("\n");
}
```
完整的转换函数会像下面这样调用:
```c
int main() {
Stack s;
convert_to_binary(15, &s);
print_binary(&s);
return 0;
}
```
阅读全文
相关推荐
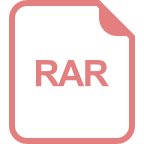















