python绘制动态折线图
时间: 2023-08-30 09:11:36 浏览: 46
你可以使用Matplotlib库来绘制动态折线图。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建一个空的折线图
fig, ax = plt.subplots()
line, = ax.plot([], [])
# 设置x轴和y轴的范围
ax.set_xlim(0, 10)
ax.set_ylim(0, 1)
# 更新折线图的数据
def update(i):
x = np.linspace(0, 10, 1000)
y = np.sin(2 * np.pi * (x - 0.01 * i))
line.set_data(x, y)
return line,
# 动画函数
ani = animation.FuncAnimation(fig, update, frames=100, interval=50, blit=True)
# 显示折线图
plt.show()
```
这个示例代码会创建一个动态的正弦曲线,你可以根据自己的需要修改数据和参数来绘制出自己想要的动态折线图。
相关问题
python绘制上下折线图
要绘制上下折线图,你可以使用matplotlib库中的fill_between函数。这个函数可以在两条折线之间填充颜色,从而形成上下折线图的效果。
首先,你需要导入matplotlib.pyplot库,并创建一个图像对象和一个坐标轴对象。然后,你可以使用plot函数绘制两条折线,分别表示上方和下方的数据。接下来,使用fill_between函数填充两条折线之间的颜色。
下面是一个示例代码,展示了如何使用matplotlib绘制上下折线图:
```python
import matplotlib.pyplot as plt
# 创建图像对象和坐标轴对象
fig, ax = plt.subplots()
# 绘制上方折线
ax.plot(game, scores, color='blue', label='上方折线')
# 绘制下方折线
ax.plot(game, \[0\]*len(scores), color='red', label='下方折线')
# 填充两条折线之间的颜色
ax.fill_between(game, scores, \[0\]*len(scores), where=(scores >= 0), interpolate=True, color='blue', alpha=0.3)
ax.fill_between(game, scores, \[0\]*len(scores), where=(scores <= 0), interpolate=True, color='red', alpha=0.3)
# 设置图例和标题
ax.legend()
ax.set_title('上下折线图')
# 显示图像
plt.show()
```
在这个示例中,我们使用了两条折线来表示上方和下方的数据。fill_between函数根据折线的正负值来填充不同的颜色,从而形成上下折线图的效果。你可以根据自己的数据和需求进行相应的修改和调整。
#### 引用[.reference_title]
- *1* [python 绘制动态折线图](https://blog.csdn.net/firefox_yau/article/details/124446088)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [Python绘制折线图](https://blog.csdn.net/qq542819222/article/details/126642243)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python绘制随机折线图
好的,以下是Python绘制随机折线图的示例代码:
```python
import random
import matplotlib.pyplot as plt
# 生成随机数据
x = [i for i in range(50)]
y = [random.randint(1, 100) for _ in range(50)]
# 绘制折线图
plt.plot(x, y)
# 设置图表标题和轴标签
plt.title('Random Line Plot')
plt.xlabel('X')
plt.ylabel('Y')
# 显示图表
plt.show()
```
在这个示例中,我们使用random模块生成了50个1到100之间的随机y坐标,并以x坐标值为0到49的整数序列作为横坐标。然后使用matplotlib库的plot函数绘制了折线图。最后,我们设置了图表标题和轴标签,并使用show函数显示图表。你可以根据需要修改代码以生成不同的随机折线图。
相关推荐
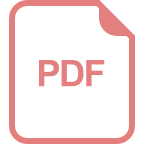
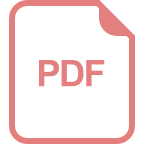












