利用Python生成坐标系随机点
时间: 2023-10-27 11:06:00 浏览: 282
可以使用Python中的random模块和matplotlib库来实现生成坐标系随机点的功能。以下是一个示例代码:
```python
import random
import matplotlib.pyplot as plt
# 生成100个随机点
x_values = [random.randint(0, 100) for _ in range(100)]
y_values = [random.randint(0, 100) for _ in range(100)]
# 绘制散点图
plt.scatter(x_values, y_values, s=10)
# 设置图表标题和坐标轴标签
plt.title("Random Points on Coordinate System", fontsize=14)
plt.xlabel("X", fontsize=10)
plt.ylabel("Y", fontsize=10)
# 设置坐标轴刻度标记的大小
plt.tick_params(axis='both', which='major', labelsize=8)
# 显示图表
plt.show()
```
这段代码会生成100个在0到100之间的随机点,并将它们绘制在坐标系上。你可以根据需要修改代码中的参数,比如点的数量、坐标轴范围等。
相关问题
利用Python生成随机点二维
生成随机点二维可以使用Python中的numpy和matplotlib库来实现。以下是一个示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 设置随机种子
np.random.seed(0)
# 生成100个二维随机点,x和y坐标均在0到1之间
points = np.random.rand(100, 2)
# 绘制散点图
plt.scatter(points[:, 0], points[:, 1], s=10)
# 设置图表标题和坐标轴标签
plt.title("Random Points in 2D Space", fontsize=14)
plt.xlabel("X", fontsize=10)
plt.ylabel("Y", fontsize=10)
# 设置坐标轴刻度标记的大小
plt.tick_params(axis='both', which='major', labelsize=8)
# 显示图表
plt.show()
```
这段代码会生成100个在二维空间内随机分布的点,并将它们绘制在坐标系上。你可以根据需要修改代码中的参数,比如点的数量、坐标轴范围等。
python创建一个笛卡尔坐标系随机坐标集数组
要使用Python创建一个表示笛卡尔坐标系中随机点的数组,可以使用`numpy`库来生成数据,并结合一些数学函数来进行坐标转换等操作。这里是一个简单的步骤指南:
### 步骤1: 安装必要的库
如果你还没有安装`numpy`, 可通过运行 `pip install numpy` 来获取它。
### 步骤2: 创建随机坐标数组
假设我们想要生成包含一定数量的二维随机坐标(x, y)。我们可以利用`numpy.random.uniform()`函数来生成均匀分布的随机数作为坐标值。
```python
import numpy as np
def create_cartesian_random_points(num_points):
"""
Create an array of random points in Cartesian coordinates.
Parameters:
num_points (int): Number of points to generate
Returns:
np.array: Array with shape (num_points, 2), where each row represents a point (x, y)
"""
# Generate random x and y values between -10 and 10 (inclusive)
x = np.random.uniform(-10, 10, size=num_points)
y = np.random.uniform(-10, 10, size=num_points)
# Combine them into the desired output format
cartesian_points = np.column_stack((x, y))
return cartesian_points
# Example usage
random_points = create_cartesian_random_points(10)
print("Random Points:")
print(random_points)
```
### 相关问题:
1. **如何调整坐标范围**? 如果需要坐标位于特定范围内(例如,[0, 5]),只需要修改`np.random.uniform()`函数的上下限即可。
2. **如何可视化这些坐标**? 使用matplotlib等绘图库可以帮助可视化生成的坐标。比如使用`scatter`函数绘制散点图。
3. **如何筛选出特定条件下的坐标**? 比如找出所有距离原点较近的点,你可以计算每个点到原点的距离并筛选符合条件的点。
通过上述步骤和示例代码,你应该能理解如何在Python中创建和处理表示笛卡尔坐标系中随机坐标集的数组了。这将为你进一步的数据分析、可视化等工作打下基础。
阅读全文
相关推荐
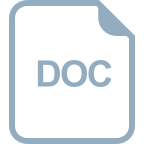
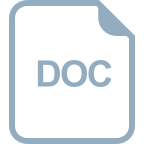
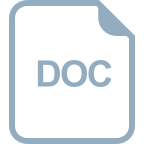


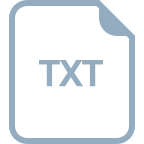
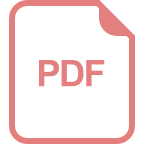
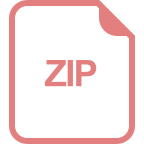
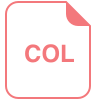







