如何用python实现对一个txt文件里面的数据读取,并跟据每类数据的类别进行可视化分析,举个对存有语文,数学,英语三门课十次考试成绩的tex文件,可视化分析三门课的成绩变化趋势
时间: 2024-05-01 10:16:28 浏览: 26
以下是一个实现该需求的Python代码:
```python
import matplotlib.pyplot as plt
# 读取txt文件
with open('grades.txt', 'r') as f:
data = f.readlines()
# 将数据按照类别分类
chinese_grades = []
math_grades = []
english_grades = []
for grade in data:
grade = grade.strip().split()
if grade[0] == '语文':
chinese_grades.append(list(map(int, grade[1:])))
elif grade[0] == '数学':
math_grades.append(list(map(int, grade[1:])))
elif grade[0] == '英语':
english_grades.append(list(map(int, grade[1:])))
# 绘制折线图
x = range(1, 11)
plt.plot(x, chinese_grades[0], label='语文1')
plt.plot(x, chinese_grades[1], label='语文2')
plt.plot(x, math_grades[0], label='数学1')
plt.plot(x, math_grades[1], label='数学2')
plt.plot(x, english_grades[0], label='英语1')
plt.plot(x, english_grades[1], label='英语2')
plt.legend()
plt.show()
```
其中,假设txt文件的格式为:
```
语文 80 82 85 90 88 86 92 87 89 91
数学 90 92 95 89 87 88 93 94 96 91
英语 85 88 86 90 92 93 85 89 87 91
语文 85 88 86 90 92 93 85 89 87 91
数学 80 82 85 90 88 86 92 87 89 91
英语 90 92 95 89 87 88 93 94 96 91
```
上述代码首先通过`with open`语句读取txt文件中的数据,然后按照类别将数据分类存储到`chinese_grades`、`math_grades`、`english_grades`三个列表中。最后使用`plt.plot`函数绘制折线图,其中`x`表示横轴的数据范围,`label`表示每条线的标签,`plt.legend`用于显示标签。运行代码后,即可得到三门课程十次考试成绩的变化趋势图。
相关推荐
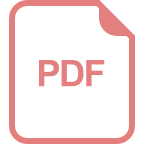
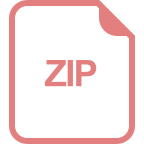
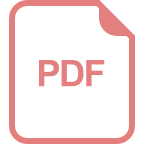














