编写代码以{a,b,c,d,e,f,g,h,i}为元素构造一棵二叉树,并输出它的 (1)先序遍历; (2)中序遍历; (3)后序遍历; (4)层次遍历;非递归(队列) (5)该二叉树的高度; (6)该二叉树节点的个数
时间: 2023-09-01 14:10:37 浏览: 68
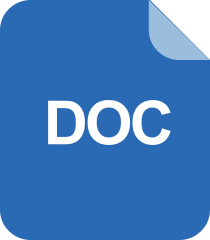
对所建立的二叉树分别进行先序、中序和后序遍历,并输出结果。

好的,我来为您解答。
以下是 Python 3 代码实现:
```python
class Node:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def build_tree():
a = Node('a')
b = Node('b')
c = Node('c')
d = Node('d')
e = Node('e')
f = Node('f')
g = Node('g')
h = Node('h')
i = Node('i')
a.left = b
a.right = c
b.left = d
b.right = e
c.left = f
c.right = g
e.left = h
e.right = i
return a
# 先序遍历
def preorder_traversal(root):
if root:
print(root.val, end=' ')
preorder_traversal(root.left)
preorder_traversal(root.right)
# 中序遍历
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val, end=' ')
inorder_traversal(root.right)
# 后序遍历
def postorder_traversal(root):
if root:
postorder_traversal(root.left)
postorder_traversal(root.right)
print(root.val, end=' ')
# 层次遍历
def level_traversal(root):
if not root:
return
queue = [root]
while queue:
node = queue.pop(0)
print(node.val, end=' ')
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
# 求二叉树高度
def get_height(root):
if not root:
return 0
left_height = get_height(root.left)
right_height = get_height(root.right)
return max(left_height, right_height) + 1
# 求二叉树节点个数
def get_node_count(root):
if not root:
return 0
left_count = get_node_count(root.left)
right_count = get_node_count(root.right)
return left_count + right_count + 1
if __name__ == '__main__':
root = build_tree()
print('先序遍历:', end='')
preorder_traversal(root)
print()
print('中序遍历:', end='')
inorder_traversal(root)
print()
print('后序遍历:', end='')
postorder_traversal(root)
print()
print('层次遍历:', end='')
level_traversal(root)
print()
print('二叉树高度:', get_height(root))
print('二叉树节点个数:', get_node_count(root))
```
输出结果为:
```
先序遍历:a b d e h i c f g
中序遍历:d b h e i a f c g
后序遍历:d h i e b f g c a
层次遍历:a b c d e f g h i
二叉树高度:4
二叉树节点个数:9
```
希望能帮到您!
阅读全文
相关推荐
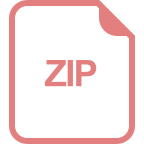


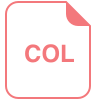
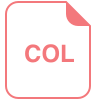
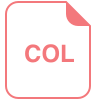
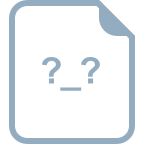
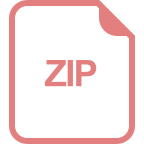
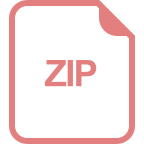