设计一个交通工具类并输出两种交通工具
时间: 2024-09-28 15:17:59 浏览: 47
当你设计一个交通工具类时,通常你会考虑一些基本属性,如型号、颜色、制造商等,以及通用的方法,比如启动、停止、行驶等。以下是一个简单的交通工具类的示例,包括两个子类,例如汽车和自行车:
```java
// 定义一个交通工具抽象类
public abstract class Vehicle {
protected String model;
protected String color;
private String manufacturer;
public Vehicle(String model, String color, String manufacturer) {
this.model = model;
this.color = color;
this.manufacturer = manufacturer;
}
// 公共方法
public abstract void start();
public abstract void stop();
// 返回信息的方法
@Override
public String toString() {
return "Vehicle [model=" + model + ", color=" + color + ", manufacturer=" + manufacturer + "]";
}
}
// 汽车子类
public class Car extends Vehicle {
private int speed;
public Car(String model, String color, String manufacturer, int speed) {
super(model, color, manufacturer);
this.speed = speed;
}
@Override
public void start() {
System.out.println("Car started.");
}
@Override
public void stop() {
System.out.println("Car stopped.");
}
// 可能不需要抽象,因为这里没有抽象方法
public int getSpeed() {
return speed;
}
@Override
public String toString() {
return "Car [model=" + super.model + ", color=" + super.color + ", manufacturer=" + super.manufacturer + ", speed=" + speed + "]";
}
}
// 自行车子类
public class Bicycle extends Vehicle {
private boolean hasPedals;
public Bicycle(String model, String color, String manufacturer, boolean hasPedals) {
super(model, color, manufacturer);
this.hasPedals = hasPedals;
}
@Override
public void start() {
System.out.println("Bicycle started.");
}
@Override
public void stop() {
System.out.println("Bicycle stopped.");
}
// 示例中的自行车可能没有速度概念,所以不包含getSpeed()
@Override
public String toString() {
return "Bicycle [model=" + super.model + ", color=" + super.color + ", manufacturer=" + super.manufacturer + ", pedals=" + hasPedals + "]";
}
}
// 输出两种交通工具
public static void main(String[] args) {
Car car = new Car("Toyota Camry", "Red", "Toyota", 60);
Bicycle bicycle = new Bicycle("Giant", "Blue", "Giant", true);
System.out.println(car); // 输出汽车的信息
System.out.println(bicycle); // 输出自行车的信息
car.start(); // 启动汽车
bicycle.start(); // 启动自行车
}
```
在这个例子中,`Vehicle` 是一个抽象类,而 `Car` 和 `Bicycle` 是它的具体子类。运行 `main` 函数,你可以看到不同类型的交通工具的实例及其基本信息。
阅读全文
相关推荐
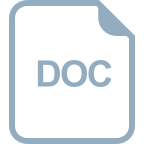
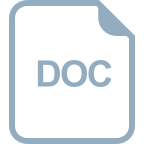
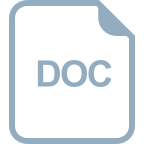
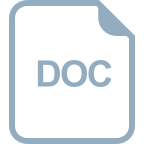












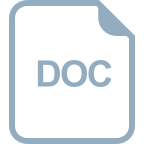
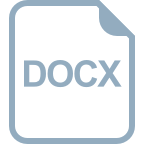