编写两个集合采用整数单链表A,B存储,设计一个算法求两个集合的并集C,C仍用单链表存储
时间: 2023-10-17 22:17:08 浏览: 92
算法思路:
1. 遍历集合A,将A中的所有元素加入集合C中;
2. 遍历集合B,对于B中的每个元素,如果该元素不在集合C中,则将它加入集合C中;
3. 返回集合C。
具体实现:
```python
class Node:
def __init__(self, val):
self.val = val
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def add(self, val):
node = Node(val)
if self.head is None:
self.head = node
else:
cur = self.head
while cur.next:
cur = cur.next
cur.next = node
def __str__(self):
if self.head is None:
return ''
cur = self.head
res = str(cur.val)
while cur.next:
cur = cur.next
res += ' -> ' + str(cur.val)
return res
def union(A, B):
C = LinkedList()
cur_A = A.head
while cur_A:
C.add(cur_A.val)
cur_A = cur_A.next
cur_B = B.head
while cur_B:
if not find(C, cur_B.val):
C.add(cur_B.val)
cur_B = cur_B.next
return C
def find(lst, val):
cur = lst.head
while cur:
if cur.val == val:
return True
cur = cur.next
return False
if __name__ == '__main__':
A = LinkedList()
A.add(1)
A.add(2)
A.add(3)
B = LinkedList()
B.add(2)
B.add(3)
B.add(4)
C = union(A, B)
print(C) # 1 -> 2 -> 3 -> 4
```
时间复杂度:$O(mn)$,其中$m$和$n$分别为集合A和B的长度。最坏情况下,B中的所有元素都不在C中,需要遍历A和B。实际上,可以通过使用哈希表等数据结构来优化查找操作,从而将时间复杂度降为$O(m+n)$。
阅读全文
相关推荐
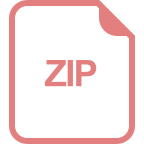


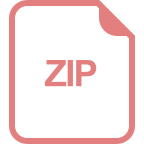
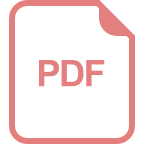
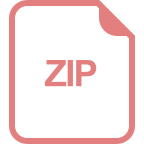
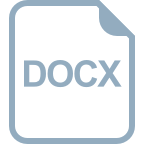
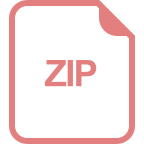