有了一张自驾旅游路线图,你会知道城市间的高速公路长度、以及该公路要收取的过路费。现在需要你写一个程序,帮助前来咨询的游客找一条出发地和目的地之间的最短路径。如果有若干条路径都是最短的,那么需要输出最便宜的一条路径。 输入格式: 输入说明:输入数据的第1行给出4个正整数N、M、S、D,其中N(2≤N≤500)是城市的个数,顺便假设城市的编号为0~(N−1);M是高速公路的条数;S是出发地的城市编号;D是目的地的城市编号。随后的M行中,每行给出一条高速公路的信息,分别是:城市1、城市2、高速公路长度、收费额,中间用空格分开,数字均为整数且不超过500。输入保证解的存在。 输出格式: 在一行里输出路径的长度和收费总额,数字间以空格分隔,输出结尾不能有多余空格。
时间: 2023-12-23 11:04:29 浏览: 150
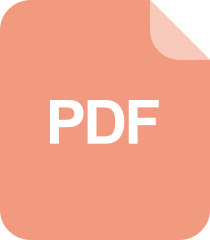
旅游高速公路智能交通系统框架探析.pdf
好的,这是一个经典的最短路径问题,可以使用Dijkstra算法来解决。以下是python实现的代码:
```python
import heapq
n, m, s, d = map(int, input().split())
# 构建邻接表
graph = [[] for _ in range(n)]
for _ in range(m):
u, v, dis, fee = map(int, input().split())
graph[u].append((v, dis, fee))
graph[v].append((u, dis, fee))
# Dijkstra算法
dist = [float('inf')] * n # 记录起点到每个点的最短距离
cost = [float('inf')] * n # 记录起点到每个点的最小花费
visited = [False] * n # 记录每个点是否已经访问
dist[s] = 0
cost[s] = 0
heap = [(0, s)] # 将起点加入堆中
while heap:
_, u = heapq.heappop(heap)
if visited[u]:
continue
visited[u] = True
for v, dis, fee in graph[u]:
if not visited[v]:
if dist[u] + dis < dist[v]: # 更新最短路径
dist[v] = dist[u] + dis
cost[v] = cost[u] + fee
heapq.heappush(heap, (dist[v], v))
elif dist[u] + dis == dist[v] and cost[u] + fee < cost[v]: # 更新最小花费
cost[v] = cost[u] + fee
heapq.heappush(heap, (dist[v], v))
print(dist[d], cost[d])
```
该算法的时间复杂度为O(mlogn),其中m为边数,n为点数。
阅读全文
相关推荐
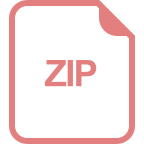

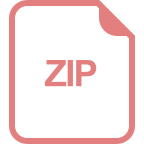
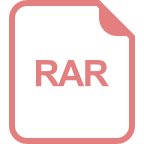
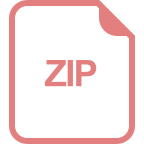
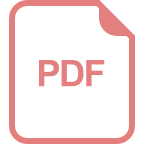
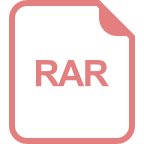
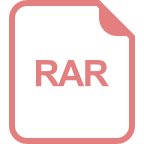
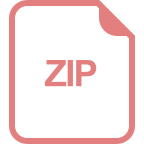
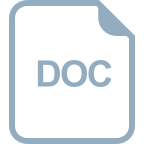
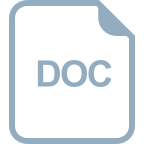
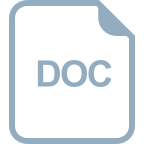
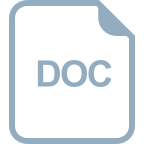
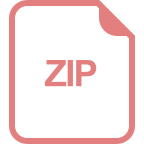
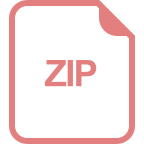