编写一个HBase的MapReduce配置程序。
时间: 2024-05-14 17:17:18 浏览: 133
好的,以下是一个简单的HBase的MapReduce配置程序的示例代码:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.io.ImmutableBytesWritable;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.mapreduce.TableReducer;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.util.GenericOptionsParser;
import java.io.IOException;
public class HBaseMapReduceExample {
public static class MyMapper extends Mapper<ImmutableBytesWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(ImmutableBytesWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] words = line.split(",");
for (String w : words) {
word.set(w);
context.write(word, one);
}
}
}
public static class MyTableReducer extends TableReducer<Text, IntWritable, ImmutableBytesWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(new ImmutableBytesWritable(Bytes.toBytes(key.toString())), new ImmutableBytesWritable(Bytes.toBytes(Integer.toString(sum))));
}
}
public static void main(String[] args) throws Exception {
Configuration conf = HBaseConfiguration.create();
String[] otherArgs = new GenericOptionsParser(conf, args).getRemainingArgs();
if (otherArgs.length != 1) {
System.err.println("Usage: HBaseMapReduceExample <tablename>");
System.exit(2);
}
String tableName = otherArgs[0];
Scan scan = new Scan();
Job job = Job.getInstance(conf, "HBaseMapReduceExample");
job.setJarByClass(HBaseMapReduceExample.class);
TableMapReduceUtil.initTableMapperJob(tableName, scan, MyMapper.class, Text.class, IntWritable.class, job);
TableMapReduceUtil.initTableReducerJob(tableName, MyTableReducer.class, job);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
这个程序实现了一个简单的词频统计功能,从 HBase 表中读取数据,将每个单词作为键,出现次数作为值,最终将结果写回 HBase 表中。
需要注意的是,程序中的 `MyMapper` 和 `MyTableReducer` 分别是 Mapper 和 Reducer 的实现类,需要根据实际场景进行修改。同时,程序中的 `tableName` 变量需要替换成实际使用的 HBase 表名。
阅读全文
相关推荐
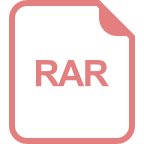
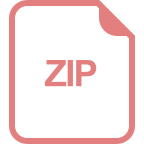
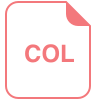

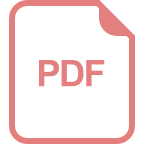
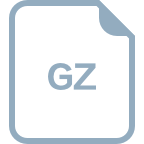
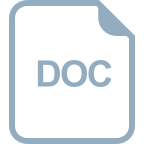
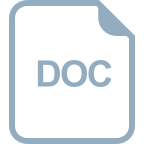
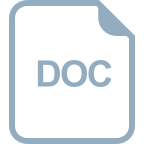
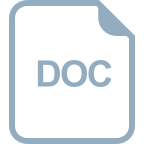
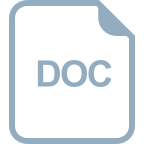
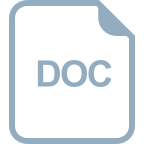
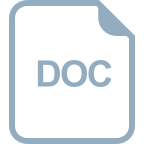
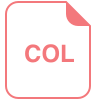
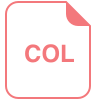