SSM框架分页展示HDFS文件列表,上传文件,删除文件,下载文件前后端代码
时间: 2023-06-12 21:05:39 浏览: 103
1. 分页展示HDFS文件列表
前端代码:
```
<template>
<div>
<el-pagination
:current-page="currentPage"
:page-size="pageSize"
:total="total"
@current-change="handleCurrentChange"
>
</el-pagination>
<el-table :data="fileList" style="width: 100%">
<el-table-column prop="fileName" label="文件名"></el-table-column>
<el-table-column prop="fileSize" label="文件大小"></el-table-column>
<el-table-column prop="owner" label="所有者"></el-table-column>
<el-table-column prop="group" label="组"></el-table-column>
<el-table-column prop="permission" label="权限"></el-table-column>
<el-table-column prop="modificationTime" label="修改时间"></el-table-column>
</el-table>
</div>
</template>
<script>
export default {
data() {
return {
fileList: [],
currentPage: 1,
pageSize: 10,
total: 0
}
},
mounted() {
this.getFileList()
},
methods: {
getFileList() {
this.$axios.get('/hdfs/file/list', {
params: {
path: '/user'
currentPage: this.currentPage,
pageSize: this.pageSize
}
}).then(res => {
if (res.success) {
this.fileList = res.data.records
this.total = res.data.total
}
})
},
handleCurrentChange(val) {
this.currentPage = val
this.getFileList()
}
}
}
</script>
```
后端代码:
```
@RequestMapping("/hdfs/file/list")
public Result<IPage<FileVO>> getFileList(String path, Integer currentPage, Integer pageSize) {
IPage<FileVO> page = new Page<>(currentPage, pageSize);
IPage<FileVO> filePage = hdfsService.getFileList(path, page);
return Result.success(filePage);
}
```
2. 上传文件
前端代码:
```
<template>
<div>
<el-upload
class="upload-demo"
:action="uploadUrl"
:headers="{ 'Content-Type': 'multipart/form-data' }"
:data="{ path: '/user' }"
:on-success="handleSuccess"
>
<el-button size="small" type="primary">点击上传</el-button>
</el-upload>
</div>
</template>
<script>
export default {
data() {
return {
uploadUrl: "/hdfs/file/upload"
}
},
methods: {
handleSuccess() {
this.$message.success("上传成功")
}
}
}
</script>
```
后端代码:
```
@PostMapping("/hdfs/file/upload")
public Result<Void> uploadFile(@RequestParam MultipartFile file, String path) {
hdfsService.uploadFile(file, path);
return Result.success();
}
```
3. 删除文件
前端代码:
```
<template>
<div>
<el-button type="danger" size="small" @click="handleDelete">删除</el-button>
</div>
</template>
<script>
export default {
props: {
row: {
type: Object,
required: true
}
},
methods: {
handleDelete() {
this.$axios.delete('/hdfs/file/delete', {
params: {
path: this.row.path
}
}).then(res => {
if (res.success) {
this.$message.success("删除成功")
this.$emit("delete-success")
}
})
}
}
}
</script>
```
后端代码:
```
@DeleteMapping("/hdfs/file/delete")
public Result<Void> deleteFile(String path) {
hdfsService.deleteFile(path);
return Result.success();
}
```
4. 下载文件
前端代码:
```
<template>
<div>
<el-button type="primary" size="small" @click="handleDownload">下载</el-button>
</div>
</template>
<script>
export default {
props: {
row: {
type: Object,
required: true
}
},
methods: {
handleDownload() {
this.$axios.get('/hdfs/file/download', {
params: {
path: this.row.path
},
responseType: 'blob'
}).then(res => {
if (res.success) {
const blob = new Blob([res.data])
const fileName = this.row.fileName
const link = document.createElement('a')
link.href = window.URL.createObjectURL(blob)
link.download = fileName
link.click()
}
})
}
}
}
</script>
```
后端代码:
```
@GetMapping("/hdfs/file/download")
public Result<byte[]> downloadFile(String path) {
byte[] bytes = hdfsService.downloadFile(path);
return Result.success(bytes);
}
```
相关推荐


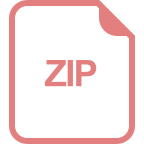
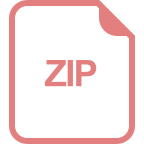
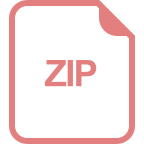
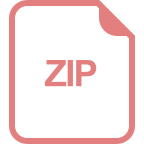
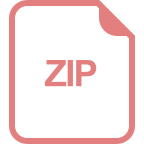