SSM框架分页展示Hdfs文件列表上传文件,删除文件,下载文件前后端代码
时间: 2023-09-15 13:05:37 浏览: 40
### 回答1:
前端代码:
```
// 分页展示Hdfs文件列表
function listFiles(pageNum) {
$.ajax({
url: "/hdfs/list",
type: "GET",
dataType: "json",
data: {"pageNum": pageNum},
success: function (result) {
var data = result.data;
var html = "";
$.each(data.list, function (index, item) {
var isDir = item.isDir ? "文件夹" : "文件";
var size = item.isDir ? "-" : (item.size / 1024).toFixed(2) + "KB";
html += "<tr><td>" + item.pathSuffix + "</td><td>" + isDir + "</td><td>" + size + "</td><td>" +
item.owner + "</td><td>" + item.group + "</td><td>" + item.permission + "</td><td>" +
item.modificationTime + "</td><td><button class='btn btn-primary' onclick='downloadFile(\"" + item.path + "\")'>下载</button>" +
"<button class='btn btn-danger' onclick='deleteFile(\"" + item.path + "\")'>删除</button></td></tr>";
});
$("#file_list").html(html);
$("#page_info").html("第 " + data.pageNum + " 页,共 " + data.pages + " 页,总 " + data.total + " 条记录");
$("#page_nav").html("<ul class='pagination'><li><a href='#' onclick='listFiles(1)'>首页</a></li>" +
"<li><a href='#' onclick='listFiles(" + data.prePage + ")'>上一页</a></li>" +
"<li><a href='#' onclick='listFiles(" + data.nextPage + ")'>下一页</a></li>" +
"<li><a href='#' onclick='listFiles(" + data.pages + ")'>末页</a></li></ul>");
}
});
}
// 上传文件
function uploadFile() {
var formData = new FormData();
formData.append("file", $("#file")[0].files[0]);
$.ajax({
url: "/hdfs/upload",
type: "POST",
data: formData,
processData: false,
contentType: false,
cache: false,
success: function (result) {
if (result.code == 200) {
alert("上传成功");
listFiles(1);
} else {
alert(result.msg);
}
}
});
}
// 删除文件
function deleteFile(path) {
if (confirm("您确认要删除该文件吗?")) {
$.ajax({
url: "/hdfs/delete",
type: "POST",
dataType: "json",
data: {"path": path},
success: function (result) {
if (result.code == 200) {
alert("删除成功");
listFiles(1);
} else {
alert(result.msg);
}
}
});
}
}
// 下载文件
function downloadFile(path) {
window.location.href = "/hdfs/download?path=" + path;
}
```
后端代码:
```
// 分页展示Hdfs文件列表
@GetMapping("/list")
@ResponseBody
public ResultDTO listFiles(@RequestParam(defaultValue = "1") Integer pageNum) {
try {
PageHelper.startPage(pageNum, 10);
List<FileStatus> fileStatuses = hdfsService.listFiles("/");
PageInfo<FileStatus> pageInfo = new PageInfo<>(fileStatuses);
Map<String, Object> data = new HashMap<>();
data.put("list", fileStatuses);
data.put("pageNum", pageInfo.getPageNum());
data.put("pages", pageInfo.getPages());
data.put("total", pageInfo.getTotal());
data.put("prePage", pageInfo.getPrePage());
data.put("nextPage", pageInfo.getNextPage());
return ResultDTO.success(data);
} catch (IOException e) {
return ResultDTO.fail("获取文件列表失败:" + e.getMessage());
}
}
// 上传文件
@PostMapping("/upload")
@ResponseBody
public ResultDTO uploadFile(@RequestParam("file") MultipartFile file) {
try {
if (file.isEmpty()) {
return ResultDTO.fail("上传文件不能为空");
}
String fileName = file.getOriginalFilename();
String filePath = "/uploads/" + fileName;
boolean result = hdfsService.uploadFile(file.getInputStream(), filePath);
if (result) {
return ResultDTO.success("上传成功");
} else {
return ResultDTO.fail("上传失败");
}
} catch (IOException e) {
return ResultDTO.fail("上传失败:" + e.getMessage());
}
}
// 删除文件
@PostMapping("/delete")
@ResponseBody
public ResultDTO deleteFile(@RequestParam String path) {
try {
boolean result = hdfsService.deleteFile(path);
if (result) {
return ResultDTO.success("删除成功");
} else {
return ResultDTO.fail("删除失败");
}
} catch (IOException e) {
return ResultDTO.fail("删除失败:" + e.getMessage());
}
}
// 下载文件
@GetMapping("/download")
@ResponseBody
public void downloadFile(@RequestParam String path, HttpServletResponse response) {
try {
InputStream inputStream = hdfsService.readFile(path);
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(path.substring(path.lastIndexOf("/") + 1), "UTF-8"));
IOUtils.copy(inputStream, response.getOutputStream());
response.flushBuffer();
} catch (IOException e) {
e.printStackTrace();
}
}
```
### 回答2:
SSM框架是指Spring + SpringMVC + MyBatis的组合,以下是一个用SSM框架实现分页展示HDFS文件列表、上传文件、删除文件、下载文件的前后端代码示例。
后端代码示例(使用Java语言编写):
1. 文件服务接口(FileService.java):
```java
public interface FileService {
List<String> getFileList(int pageNum, int pageSize);
void uploadFile(MultipartFile file);
void deleteFile(String filename);
void downloadFile(String filename, HttpServletResponse response);
}
```
2. 文件服务实现类(FileServiceImpl.java):
```java
@Service
public class FileServiceImpl implements FileService {
private static final String HDFS_PATH = "hdfs://localhost:9000/";
@Override
public List<String> getFileList(int pageNum, int pageSize) {
List<String> fileList = new ArrayList<>();
try {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(URI.create(HDFS_PATH), conf);
RemoteIterator<LocatedFileStatus> it = fs.listFiles(new Path(HDFS_PATH), true);
// 跳过前pageNum*pageSize个文件
for (int i = 0; i < pageNum * pageSize; i++) {
if (it.hasNext()) {
it.next();
} else {
break;
}
}
// 获取接下来的pageSize个文件路径
for (int i = 0; i < pageSize; i++) {
if (it.hasNext()) {
fileList.add(it.next().getPath().toString());
} else {
break;
}
}
fs.close();
} catch (IOException e) {
e.printStackTrace();
}
return fileList;
}
@Override
public void uploadFile(MultipartFile file) {
try {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(URI.create(HDFS_PATH), conf);
fs.copyFromLocalFile(new Path(file.getOriginalFilename()), new Path(HDFS_PATH));
fs.close();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void deleteFile(String filename) {
try {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(URI.create(HDFS_PATH), conf);
fs.delete(new Path(HDFS_PATH + filename), true);
fs.close();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void downloadFile(String filename, HttpServletResponse response) {
try {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(URI.create(HDFS_PATH), conf);
response.setHeader("Content-Disposition", "attachment; filename=\"" + filename + "\"");
response.setContentType("application/octet-stream");
IOUtils.copyBytes(fs.open(new Path(HDFS_PATH + filename)), response.getOutputStream(), conf);
fs.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
3. 控制器类(FileController.java):
```java
@RestController
@RequestMapping("/file")
public class FileController {
@Autowired
private FileService fileService;
@GetMapping("/list")
public List<String> getFileList(@RequestParam("pageNum") int pageNum, @RequestParam("pageSize") int pageSize) {
return fileService.getFileList(pageNum, pageSize);
}
@PostMapping("/upload")
public void uploadFile(@RequestParam("file") MultipartFile file) {
fileService.uploadFile(file);
}
@DeleteMapping("/delete")
public void deleteFile(@RequestParam("filename") String filename) {
fileService.deleteFile(filename);
}
@GetMapping("/download")
public void downloadFile(@RequestParam("filename") String filename, HttpServletResponse response) {
fileService.downloadFile(filename, response);
}
}
```
前端代码示例:
页面展示文件列表、上传文件、删除文件使用HTML和JavaScript实现,其中API请求使用jQuery的`ajax`方法。
```html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="utf-8">
<title>HDFS文件管理</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>HDFS文件管理</h1>
<h2>文件列表</h2>
<div id="fileList"></div>
<h2>上传文件</h2>
<input type="file" id="fileInput">
<button type="button" onclick="uploadFile()">上传</button>
<h2>删除文件</h2>
<input type="text" id="filenameInput" placeholder="文件名">
<button type="button" onclick="deleteFile()">删除</button>
<script>
// 获取文件列表
function getFileList(pageNum, pageSize) {
$.ajax({
url: "/file/list",
type: "GET",
data: {
pageNum: pageNum,
pageSize: pageSize
},
success: function (data) {
var fileList = "";
for (var i = 0; i < data.length; i++) {
fileList += "<p>" + data[i] + "</p>";
}
$("#fileList").html(fileList);
},
error: function () {
alert("获取文件列表失败");
}
});
}
// 上传文件
function uploadFile() {
var fileInput = document.getElementById('fileInput');
var file = fileInput.files[0];
var formData = new FormData();
formData.append("file", file);
$.ajax({
url: "/file/upload",
type: "POST",
data: formData,
processData: false,
contentType: false,
success: function () {
alert("上传成功");
getFileList(1, 10); // 刷新文件列表
},
error: function () {
alert("上传失败");
}
});
}
// 删除文件
function deleteFile() {
var filenameInput = document.getElementById('filenameInput');
var filename = filenameInput.value;
$.ajax({
url: "/file/delete",
type: "DELETE",
data: {
filename: filename
},
success: function () {
alert("删除成功");
getFileList(1, 10); // 刷新文件列表
},
error: function () {
alert("删除失败");
}
});
}
getFileList(1, 10); // 页面加载时获取文件列表
</script>
</body>
</html>
```
以上是一个使用SSM框架实现分页展示HDFS文件列表、上传文件、删除文件、下载文件的前后端代码示例。请根据实际需求进行适当修改和补充。
### 回答3:
SSM框架通常用于开发JavaWeb项目,其中S为Spring、S为SpringMVC、M为MyBatis。下面将介绍如何使用SSM框架进行HDFS文件列表的分页展示、文件的上传、删除和下载的前后端代码。
1. 后端Java代码:
- 定义文件列表的数据模型:
```
public class HdfsFile {
private String name;
private String path;
// get、set方法省略
}
```
- 编写文件列表的服务类:
```
@Service
public class HdfsFileService {
@Autowired
private FileSystem fs;
public List<HdfsFile> getFileList(String path, int pageNum, int pageSize) throws IOException {
List<HdfsFile> fileList = new ArrayList<>();
// 使用HDFS API获取指定路径下的文件列表,可通过FileSystem类的globStatus或listFiles方法实现
// 将获取的文件列表转换为HdfsFile对象,设置name和path属性
// 根据pageNum和pageSize进行分页处理
// 返回分页结果
return fileList;
}
public void uploadFile(String localPath, String hdfsPath) throws IOException {
// 使用HDFS API将本地文件上传至HDFS,可通过FileSystem类的copyFromLocalFile方法实现
}
public void deleteFile(String hdfsPath) throws IOException {
// 使用HDFS API删除HDFS中的文件,可通过FileSystem类的delete方法实现
}
public void downloadFile(String hdfsPath, String localPath) throws IOException {
// 使用HDFS API从HDFS下载文件到本地,可通过FileSystem类的copyToLocalFile方法实现
}
}
```
- 编写后端Controller类:
```
@Controller
@RequestMapping("/hdfs")
public class HdfsFileController {
@Autowired
private HdfsFileService hdfsFileService;
@RequestMapping("/list")
@ResponseBody
public List<HdfsFile> getFileList(String path, int pageNum, int pageSize) throws IOException {
return hdfsFileService.getFileList(path, pageNum, pageSize);
}
@RequestMapping("/upload")
@ResponseBody
public void uploadFile(String localPath, String hdfsPath) throws IOException {
hdfsFileService.uploadFile(localPath, hdfsPath);
}
@RequestMapping("/delete")
@ResponseBody
public void deleteFile(String hdfsPath) throws IOException {
hdfsFileService.deleteFile(hdfsPath);
}
@RequestMapping("/download")
@ResponseBody
public void downloadFile(String hdfsPath, String localPath) throws IOException {
hdfsFileService.downloadFile(hdfsPath, localPath);
}
}
```
2. 前端代码:
- 分页展示HDFS文件列表的页面:
```
<!DOCTYPE html>
<html>
<head>
<title>HDFS文件列表</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</head>
<body>
<div id="fileListContainer"></div>
<script>
var pageNum = 1;
var pageSize = 10;
function getFileList() {
$.ajax({
url: "/hdfs/list",
type: "get",
data: {
path: "/",
pageNum: pageNum,
pageSize: pageSize
},
success: function (data) {
var fileListContainer = $("#fileListContainer");
fileListContainer.empty();
for (var i = 0; i < data.length; i++) {
var file = data[i];
fileListContainer.append("<div>" + file.name + "</div>");
}
}
});
}
$(document).ready(function () {
getFileList();
});
</script>
</body>
</html>
```
- 上传文件的页面:
```
<!DOCTYPE html>
<html>
<head>
<title>上传文件</title>
</head>
<body>
<input type="file" id="fileInput">
<button onclick="uploadFile()">上传</button>
<script>
function uploadFile() {
var fileInput = document.getElementById("fileInput");
var file = fileInput.files[0];
var formData = new FormData();
formData.append("file", file);
$.ajax({
url: "/hdfs/upload",
type: "post",
data: formData,
processData: false,
contentType: false,
success: function () {
alert("文件上传成功");
}
});
}
</script>
</body>
</html>
```
- 删除文件的页面:
```
<!DOCTYPE html>
<html>
<head>
<title>删除文件</title>
</head>
<body>
<input type="text" id="hdfsPathInput">
<button onclick="deleteFile()">删除</button>
<script>
function deleteFile() {
var hdfsPath = document.getElementById("hdfsPathInput").value;
$.ajax({
url: "/hdfs/delete",
type: "post",
data: {
hdfsPath: hdfsPath
},
success: function () {
alert("文件删除成功");
}
});
}
</script>
</body>
</html>
```
- 下载文件的页面:
```
<!DOCTYPE html>
<html>
<head>
<title>下载文件</title>
</head>
<body>
<input type="text" id="hdfsPathInput">
<input type="text" id="localPathInput">
<button onclick="downloadFile()">下载</button>
<script>
function downloadFile() {
var hdfsPath = document.getElementById("hdfsPathInput").value;
var localPath = document.getElementById("localPathInput").value;
$.ajax({
url: "/hdfs/download",
type: "post",
data: {
hdfsPath: hdfsPath,
localPath: localPath
},
success: function () {
alert("文件下载成功");
}
});
}
</script>
</body>
</html>
```
以上就是使用SSM框架进行HDFS文件列表的分页展示、上传、删除和下载的前后端代码。具体实现还需要根据实际情况进行调整和完善。
相关推荐
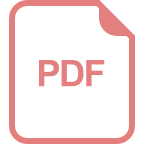
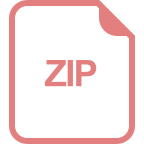
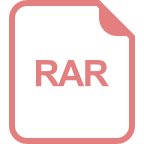


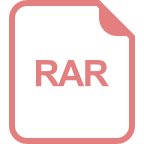
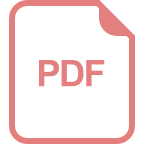
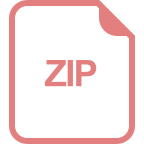
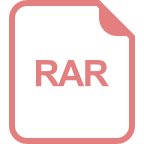
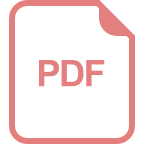
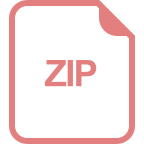
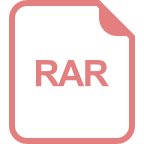
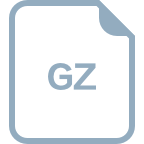
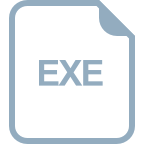