用python实现语音识别功能_语音/文本互相转换
时间: 2024-10-11 11:07:12 浏览: 82
在Python中实现语音识别功能通常通过集成第三方库,如Google的SpeechRecognition模块和阿里云的智能语音服务。以下是基本步骤:
1. 安装所需库:
使用pip安装` SpeechRecognition` 和 `pydub`(用于处理音频文件),以及根据需要阿里云的SDK。
```bash
pip install SpeechRecognition pydub aliyun-python-sdk-aip
```
2. Google语音识别示例(基于Web API):
```python
import speech_recognition as sr
r = sr.Recognizer()
with sr.AudioFile('path_to_your_audio.wav') as source:
audio_data = r.record(source)
try:
text = r.recognize_google(audio_data, language='zh-CN')
print(f"你说的是: {text}")
except sr.UnknownValueError:
print("无法理解音频")
except sr.RequestError as e:
print(f"请求错误; {e}")
```
3. 阿里云ASR(自动语音识别)示例(需要API Key):
```python
import aip
client = aip.AipSpeech(
'your_client_id', 'your_client_secret', 'your_instance_id'
)
# 将语音转成文字
def transcribe(file_path):
with open(file_path, 'rb') as f:
result = client.asr(f.read(), {'format': 'wav', 'language': 'zh'})
return result['result'][0]
audio_text = transcribe('path_to_your_audio.wav')
print(f"语音内容:{audio_text}")
4. 文本转语音:
可以使用`gTTS`(Google Text-to-Speech)库将文本转换为MP3或WAV音频文件。
```python
from gtts import gTTS
tts = gTTS(text="你好,这是文本转语音", lang='zh-cn')
tts.save("output.mp3")
```
阅读全文
相关推荐
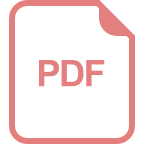
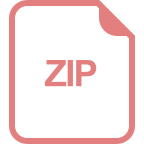
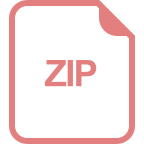

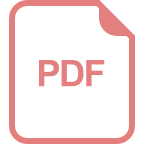
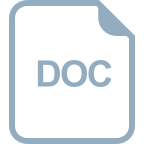
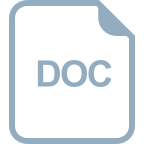
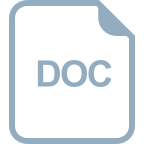
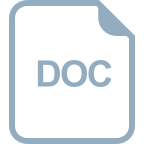
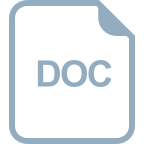
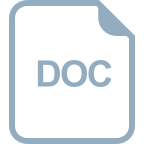
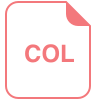
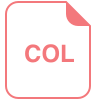
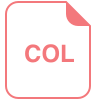
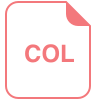
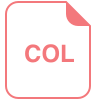
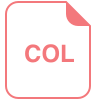