pthread创建多线程
时间: 2023-08-20 22:04:33 浏览: 117
要在C语言中创建多线程,可以使用pthread库。下面是一个示例代码,演示了如何使用pthread库创建多个线程:
```c
#include <stdio.h>
#include <pthread.h>
#define NUM_THREADS 5
// 线程函数
void* threadFunc(void* arg) {
long thread_id = (long)arg;
printf("Thread %ld is running\n", thread_id);
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int rc;
long t;
// 创建多个线程
for (t = 0; t < NUM_THREADS; t++) {
printf("Creating thread %ld\n", t);
rc = pthread_create(&threads[t], NULL, threadFunc, (void*)t);
if (rc) {
printf("Error: return code from pthread_create() is %d\n", rc);
return -1;
}
}
// 等待所有线程结束
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[t], NULL);
}
printf("All threads are finished\n");
return 0;
}
```
在上面的示例中,我们使用了`pthread_create`函数来创建多个线程。每个线程都运行相同的`threadFunc`函数,并打印出线程的ID。使用`pthread_join`函数等待所有线程完成后,程序最后打印"All threads are finished"。
请注意,在编译时需要链接pthread库,可以使用以下命令进行编译:
```
gcc -o program program.c -pthread
```
这样就可以创建并运行多个线程了。
阅读全文
相关推荐


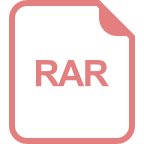
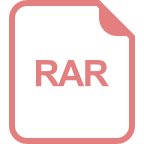









